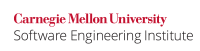
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <Windows.h> #include <wincrypt.h> #include <stdio.h> void func(void) { HCRYPTPROV prov; if (CryptAcquireContext(&prov, NULL, NULL, PROV_RSA_FULL, 0)) { long int li = 0; if (CryptGenRandom(prov, sizeof(li), (BYTE *)&li)) { printf("Random number: %ld\n", li); } else { /* Handle error */ } if (!CryptReleaseContext(prov, 0)) { /* Handle error */ } } else { /* Handle error */ } } |
Compliant Solution (Windows)
On Windows platforms, the rand_s()
function can be used to generate cryptographically strong random numbers.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h>
#include <stdlib.h>
int func(void) {
unsigned int number;
errno_t err;
err = rand_s( &number);
if (err != 0) {
/* handle error */
} else {
printf("Random number: %u\n", number)
}
} |
Risk Assessment
The use of the rand()
function can result in predictable random numbers.
...