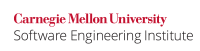
Comments and Contributing
We provide access to the community members to contribute after subject matter expertise is verified.
If you want to provide a suggestion or comment and do not have an account, please submit feedback.
If you have an account but are having problems with access, also please submit a support request.
Accounts are regularly deactivated after extended periods of inactivity.
Rules
Back Matter
Contact Us
Contact us if you
- have questions about the Secure Coding wiki
- have recommendations for standards in development
- want to request privileges to participate in standards development
Thank You!
We acknowledge the contributions of the following folks, and we look forward to seeing your name here as well.
3 Comments
thiago glauco sanchez
Like others languages, is not a good idea compare floating point numbers in Perl. For solving that you can:
transform the numbers in strings and compare the strings according hte precision needs of yours:
sub are_equals {
#dont forget to sanitize $num1 and $num2 before using into sprintf
my ($num1, $num2, $precision) = @_;
return sprintf("%.${precision}f", $num1) eq sprintf("%.${precision}f", $num2);
}
define an acceptable error range ($delta) for your project:
sub are_equals {
my ($num1, $num2, $delta) = @_;
if ( $delta >= ($num1 - $num2) )
return 1;
return 0; }
Or just use bignum accordingly.
David Svoboda
Yes, there is a world of information on how to handle floating-point arithmetic. IIRC the Perl FAQ has some info. Also CERT has plenty of info on their C wiki: Rule 05. Floating Point (FLP). Since most languages use IEEE 754 for fp arithmetic, mostly the do's and dont's are language-independent
Anonymous
You'll have troubles if $num1 is "too much" less than $num2 (think calling are_equals(0, 2, 1)).
abs() helps here:
sub are_equals {
my ($num1, $num2, $delta) = @_;
if ( $delta >= abs($num1 - $num2) )
return 1;
return 0; }
I also find it more readable to put the difference in the left hand side and call $delta with a more expressive (IMHO) name:
sub are_equals {
my ($num1, $num2, $max_delta) = @_;
return abs($num1 - $num2) <= $max_delta;
}