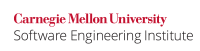
...
When GCC 3.4.6 compiles this code with optimization, the assignment through the aliased pointer is effectively eliminated.
Compliant Solution
...
This compliant solution uses a union
type that includes a type compatible with the effective type of the object:
...
The printf()
typically outputs "2222 2222". However, there is no guarantee that this will be true; the object representations of a
and i
are unspecified and need not be compatible in this way, despite this operation being commonly accepted as an implementation extension. (See unspecified behavior 11.)
Compliant Solution (memcpy)
This compliant solution uses memcpy
to convert the short
s to an int
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h>
#include <string.h>
void func(void) {
short a[2];
a[0]=0x1111;
a[1]=0x1111;
int i = 0x22222222;
memcpy(a, &i, sizeof a);
printf("%x %x\n", a[0], a[1]);
} |
A good optimizing compiler will recognize the use of memcpy
and will emit code just as fast as the union
approach.
Noncompliant Code Example
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> struct gadget { int i; double d; char *p; }; struct widget { char *q; int j; double e; }; void func(void) { struct gadget *gp; struct widget *wp; gp = (struct gadget *)malloc(sizeof(struct gadget)); if (!gp) { /* Handle error */ } /* ... Initialize gadget ... */ wp = (struct widget *)realloc(gp, sizeof(struct widget)); if (!wp) { free(gp); /* Handle error */ } if (wp->j == 12) { /* ... */ } /* ... */ free(wp); } |
Compliant Solution
This compliant solution reuses the memory from the gadget
object but reinitializes the memory to a consistent state before reading from it:
...
Most compilers will produce a warning diagnostic if the two array types used in an expression are incompatible.
Compliant Solution
In this compliant solution, b
is declared to point to an array with the same number of elements as a
, satisfying the size specifier criterion for array compatibility:
...