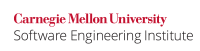
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h> void f(signed int si_a, signed int si_b) { signed int sum; if (((si_b > 0) && (si_a > (INT_MAX - si_b))) || ((si_b < 0) && (si_a < (INT_MIN - si_b)))) { /* Handle error */ } else { sum = si_a + si_b; } /* ... */ } |
Subtraction
Subtraction is between two operands of arithmetic type, two pointers to qualified or unqualified versions of compatible object types, or a pointer to an object type and an integer type. This rule applies only to subtraction between two operands of arithmetic type. (See ARR36-C. Do not subtract or compare two pointers that do not refer to the same array, ARR37-C. Do not add or subtract an integer to a pointer to a non-array object, and ARR30-C. Do not form or use out-of-bounds pointers or array subscripts for information about pointer subtraction.)
Decrementing is equivalent to subtracting 1.
Noncompliant Code Example
This noncompliant code example can result in a signed integer overflow during the subtraction of the signed operands si_a
and si_b
:
Compliant Solution (GNU)
This compliant solution uses the GNU extension __builtin_sadd_overflow
, available with GCC and Clang:
Code Block | ||||
---|---|---|---|---|
| ||||
void f(signed int si_a, signed int si_b) {
signed int sum;
if (__builtin_sadd_overflow(si_a, si_b, &sum)) {
/* Handle error */
}
/* ... */
} |
Subtraction
Subtraction is between two operands of arithmetic type, two pointers to qualified or unqualified versions of compatible object types, or a pointer to an object type and an integer type. This rule applies only to subtraction between two operands of arithmetic type. (See ARR36-C. Do not subtract or compare two pointers that do not refer to the same array, ARR37-C. Do not add or subtract an integer to a pointer to a non-array object, and ARR30-C. Do not form or use out-of-bounds pointers or array subscripts for information about pointer subtraction.)
Decrementing is equivalent to subtracting 1.
Noncompliant Code Example
This noncompliant code example can result in a signed integer overflow during the subtraction of the signed operands si_a
and si_b
:
Code Block | ||||
---|---|---|---|---|
| ||||
void func(signed int si_a, signed int si_b) {
signed int diff = si_a - si_b;
/* ... */
} |
Compliant Solution
This compliant solution tests the operands of the subtraction to guarantee there is no possibility of signed overflow, regardless of representation:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h>
void func(signed int si_a, signed int si_b) {
signed int diff;
if ((si_b > 0 && si_a < INT_MIN + si_b) ||
(si_b < 0 && si_a > INT_MAX + si_b)) {
/* Handle error */
} else {
| ||||
Code Block | ||||
| ||||
void func(signed int si_a, signed int si_b) { signed int diff = si_a - si_b; } /* ... */ } |
Compliant Solution (GNU)
This compliant solution tests the operands of the subtraction to guarantee there is no possibility of signed overflow, regardless of representationuses the GNU extension __builtin_ssub_overflow
, available with GCC and Clang:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h> void func(signed int si_a, signed int si_b) { signed int diff; if ((si_b > 0 && int si_a, < INT_MIN +signed int si_b) ||{ signed int diff; if (si_b < 0 && __builtin_ssub_overflow(si_a > INT_MAX + , si_b, &diff)) { /* Handle error */ } else { diff = si_a - si_b; */ } /* ... */ } |
Multiplication
...
The assertion fails if long long
has less than twice the precision of int
. The PRECISION()
macro and popcount()
function provide the correct precision for any integer type. (See INT35-C. Use correct integer precisions.)
Compliant Solution
The following portable compliant solution can be used with any conforming implementation, including those that do not have an integer type that is at least twice the precision of int
:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h> void func(signed int si_a, signed int si_b) { signed int result; if (si_a > 0) { /* si_a is positive */ if (si_b > 0) { /* si_a and si_b are positive */ if (si_a > (INT_MAX / si_b)) { /* Handle error */ } } else { /* si_a positive, si_b nonpositive */ if (si_b < (INT_MIN / si_a)) { /* Handle error */ } } /* si_a positive, si_b nonpositive */ } else { /* si_a is nonpositive */ if (si_b > 0) { /* si_a is nonpositive, si_b is positive */ if (si_a < (INT_MIN / si_b)) { /* Handle error */ } } else { /* si_a and si_b are nonpositive */ if ( (si_a != 0) && (si_b < (INT_MAX / si_a))) { /* Handle error */ } } /* End if si_a and si_b are nonpositive */ } /* End if si_a is nonpositive */ result = si_a * si_b; (INT_MAX / si_a))) { /* Handle error */ } } /* End if si_a and si_b are nonpositive */ } /* End if si_a is nonpositive */ result = si_a * si_b; } |
Compliant Solution (GNU)
This compliant solution uses the GNU extension __builtin_smul_overflow
, available with GCC and Clang:
Code Block | ||||
---|---|---|---|---|
| ||||
void func(signed int si_a, signed int si_b) {
signed int result;
if (__builtin_smul_overflow(si_a, si_b, &result)) {
/* Handle error */
}
} |
Division
Division is between two operands of arithmetic type. Overflow can occur during two's complement signed integer division when the dividend is equal to the minimum (negative) value for the signed integer type and the divisor is equal to −1
. Division operations are also susceptible to divide-by-zero errors. (See INT33-C. Ensure that division and remainder operations do not result in divide-by-zero errors.)
...
The C Standard, 6.5.7, paragraph 4 [ISO/IEC 9899:2011], states
...
In almost every case, an attempt to shift by a negative number of bits or by more bits than exist in the operand indicates a logic error. These issues are covered by INT34-C. Do not shift an expression by a negative number of bits or by greater than or equal to the number of bits that exist in the operand.
Noncompliant Code Example
This noncompliant code example performs a left shift, after verifying that the number being shifted is not negative, and the number of bits to shift is valid. The PRECISION()
macro and popcount()
function provide the correct precision for any integer type. (See INT35-C. Use correct integer precisions.) However, because this code does no overflow check, it can result in an unrepresentable value.
...
Compliant Solution
This compliant solution eliminates the possibility of overflow resulting from a left-shift operation:
...