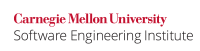
Signed integer overflow is undefined behavior 36. Consequently, implementations have considerable latitude in how they deal with signed signede integer overflow. (See MSC15-C. Do not depend on undefined behavior.) An implementation that defines signed integer types as being modulo, for example, need not detect integer overflow. Implementations may also trap on signed arithmetic overflows, or simply assume that overflows will never happen and generate object code accordingly. It is also possible for the same conforming implementation to emit code that exhibits different behavior in different contexts. For example, an implementation may determine that a signed integer loop control variable declared in a local scope cannot overflow and may emit efficient code on the basis of that determination, while the same implementation may determine that a global variable used in a similar context will wrap.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h> void f(signed int si_a, signed int si_b) { signed int sum; if (((si_b > 0) && (si_a > (INT_MAX - si_b))) || ((si_b < 0) && (si_a < (INT_MIN - si_b)))) { /* Handle error */ } else { sum = si_a + si_b; } /* ... */ } |
Compliant Solution (
...
Glibc)
This compliant solution uses the GNU extension __builtin_sadd_overflow
, available with GCC, Clang, and ICC:
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h> void func(signed int si_a, signed int si_b) { signed int diff; if ((si_b > 0 && si_a < INT_MIN + si_b) || (si_b < 0 && si_a > INT_MAX + si_b)) { /* Handle error */ } else { diff = si_a - si_b; } /* ... */ } |
Compliant Solution (
...
Glibc)
This compliant solution uses the GNU extension __builtin_ssub_overflow
, available with GCC, Clang, and ICC:
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <limits.h> void func(signed int si_a, signed int si_b) { signed int result; if (si_a > 0) { /* si_a is positive */ if (si_b > 0) { /* si_a and si_b are positive */ if (si_a > (INT_MAX / si_b)) { /* Handle error */ } } else { /* si_a positive, si_b nonpositive */ if (si_b < (INT_MIN / si_a)) { /* Handle error */ } } /* si_a positive, si_b nonpositive */ } else { /* si_a is nonpositive */ if (si_b > 0) { /* si_a is nonpositive, si_b is positive */ if (si_a < (INT_MIN / si_b)) { /* Handle error */ } } else { /* si_a and si_b are nonpositive */ if ( (si_a != 0) && (si_b < (INT_MAX / si_a))) { /* Handle error */ } } /* End if si_a and si_b are nonpositive */ } /* End if si_a is nonpositive */ result = si_a * si_b; } |
Compliant Solution (
...
Glibc)
This compliant solution uses the GNU extension __builtin_smul_overflow
, available with GCC, Clang, and ICC:
...
The C Standard, 6.5.7, paragraph 4 [ISO/IEC 9899:2011], states
...
In almost every case, an attempt to shift by a negative number of bits or by more bits than exist in the operand indicates a logic error. These issues are covered by INT34-C. Do not shift an expression by a negative number of bits or by greater than or equal to the number of bits that exist in the operand.
Noncompliant Code Example
This noncompliant code example performs a left shift, after verifying that the number being shifted is not negative, and the number of bits to shift is valid. The PRECISION()
macro and popcount()
function provide the correct precision for any integer type. (See INT35-C. Use correct integer precisions.) However, because this code does no overflow check, it can result in an unrepresentable value.
...
Compliant Solution
This compliant solution eliminates the possibility of overflow resulting from a left-shift operation:
...