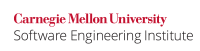
...
Code Block | ||||
---|---|---|---|---|
| ||||
LinkedList bannedUsers; int is_banned(User usr) { int x = 0; Node cur_node = (bannedUsers->head); while (cur_node != NULL) { if(!strcmp((char *)cur_node->data, usr->name)) { x++; } cur_node = cur_node->next; } return x; } void processRequest(User usr) { if(is_banned(usr) == 1) { return; } serveResults(); } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
LinkedList bannedUsers; int is_banned(User usr) { int x = 0; Node cur_node = (bannedUsers->head); while(cur_node != NULL) { if (!strcmp((char *)cur_node->data, usr->name)) { x++; } cur_node = cur_node->next; } return x; } void processRequest(User usr) { if (is_banned(usr) != 0) { return; } serveResults(); } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
void login(char *usr, char *pw) {
User user = find_user(usr);
if (!strcmp((user->password),pw_given)) {
grantAccess();
}
denyAccess("Incorrect Password");
}
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
void login(char *usr, char *pw) {
User user = find_user(usr);
if (strcmp((user->password),pw_given) == 0) {
grantAccess();
}
denyAccess("Incorrect Password");
}
|
...