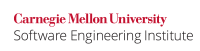
...
For assertions involving only constant expressions, some implementations allow the use of a preprocessor conditional statement may be used, as in this example:
Code Block | ||||
---|---|---|---|---|
| ||||
struct timer { unsigned char MODE; unsigned int DATA; unsigned int COUNT; }; #if (sizeof(struct timer) == sizeof(unsigned char) + sizeof(unsigned int) + sizeof(unsigned int)) #error "Structure must not have any padding" #endif |
Using #error
directives allows for clear diagnostic messages. Because this approach evaluates assertions at compile time, there is no runtime penalty.
Unfortunately, this solution is not portable. The C Standard does not require that implementations support sizeof
, offsetof
, or enumeration constants in #if
conditions. According to section 6.10.1, "Conditional inclusion," all identifiers in the expression that controls conditional inclusion either are or are not macro names. Some compilers allow these constructs in conditionals as an extension, but most do not.
Compliant Solution
This portable compliant solution uses static_assert
.
Code Block | ||||
---|---|---|---|---|
| ||||
struct timer {
unsigned char MODE;
unsigned int DATA;
unsigned int COUNT;
};
#if (sizeof(struct timer) == sizeof(unsigned char) + sizeof(unsigned int) + sizeof(unsigned int))
#error "Structure must not have any padding"
#endif
static_assert(sizeof(struct timer) == sizeof(unsigned char) + sizeof(unsigned int) + sizeof(unsigned int),
"Structure must not have any padding");
|
...