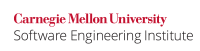
...
Code Block | ||||
---|---|---|---|---|
| ||||
void login(char *usr, char *pw) { User user = find_user(usr); if (!strcmp((user->password),pw)) { grantAccess(); } else { denyAccess("Incorrect Password"); } } |
The preceding code works correctly. However, to simplify the login code or to facilitate checking a user's password more than once, a programmer can separate the password-checking code from the login function in the following way:
Code Block | ||||
---|---|---|---|---|
| ||||
int check_password(User *user, char *pw_given) { if (!strcmp((user->password),pw_given)) { return 1; } return 0; } void login(char *usr, char *pw) { User user = find_user(usr); if (!check_password(user, pw)) { grantAccess(); } else { denyAccess("Incorrect Password"); } } |
In an attempt to leave the previous logic intact, the developer just replaces strcmp()
with a call to the new function. However, doing so produces incorrect behavior. In this case, any user who inputs an incorrect password is granted access. Again, two conventions conflict and produce code that is easily corrupted when modified. To make code maintainable and to avoid these conflicts, such a result should never be defaulted.
...
Code Block | ||||
---|---|---|---|---|
| ||||
void login(char *usr, char *pw) { User user = find_user(usr); if (strcmp((user->password),pw) == 0) { grantAccess(); } else { denyAccess("Incorrect Password"); } } |
Risk Assessment
Code that does not conform to the common practices presented is difficult to maintain. Bugs can easily arise when modifying helper functions that evaluate true/false or success/failure. Bugs can also easily arise when modifying code that tests for equality using a comparison function that obeys the same conventions as standard library functions such as strcmp
.
...