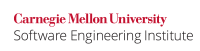
The logical AND and logical OR operators (&&
and ||
, respectively) exhibit "short-circuit" operation. That is, the second operand is not evaluated if the result can be deduced solely by evaluating the first operand.
One Programmers should exercise caution if the second operand contains side effects because it may not be apparent whether the side effects actually occur.
In the following code, the value of i
is incremented only when i >= 0
.
Code Block |
---|
enum { max = 15 };
int i = /* initialize to user supplied value */;
if ( (i >= 0) && ( (i++) <= max) ) {
/* code */
}
|
Although the behavior is well defined, it is not immediately obvious whether or not i
gets incremented or not.
Noncompliant Code Example
...
Code Block | ||||
---|---|---|---|---|
| ||||
char *p = /* initialize, may or may not be NULL */
if (p || (p = (char *) malloc(BUF_SIZE)) ) {
/* do stuff with p */
free(p);
p = NULL;
}
else {
/* handle malloc() error */
return;
}
|
Because malloc()
is only called if p
is NULL
when entering the if
clause, free()
might be called with a pointer to local data not allocated by malloc()
. (See rule MEM34-C. Only free memory allocated dynamically.) This is partially due to the uncertainty of whether or not malloc()
is actually called or not.
Compliant Solution
In this compliant solution, a second pointer, q
, is used to indicate whether malloc()
is called; if not, q
remains set to NULL
. Passing NULL
to free()
is guaranteed to safely do nothing.
Code Block | ||||
---|---|---|---|---|
| ||||
char *p;
char *q = NULL;
if (p == NULL) {
q = (char *) malloc(BUF_SIZE);
p = q;
}
if (p == NULL) {
/* handle malloc() error */
return;
}
/* do stuff with p */
free(q);
q = NULL;
|
...
Tool | Version | Checker | Description | section|||||||
---|---|---|---|---|---|---|---|---|---|---|
| Section | 35 D | Fully Implementedimplemented. | |||||||
Section | Compass/ROSE |
|
| Section | Could detect possible violations of this recommendation by reporting expressions with side effects, including function calls, that appear on the right-hand-side of an |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...
MITRE CWE: CWE-768, "Incorrect Short Circuit Evaluation"
ISO/IEC 9899:19992011 Section 6.5.13, "Logical AND operator," and Section 6.5.14, "Logical OR operator"
...