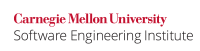
Signed integer overflow is undefined behavior (see undefined behavior 36 in Annex J of C11 [ISO/IEC 9899:2011]). This means that implementations have a great deal of latitude in how they deal with signed integer overflow.
...
For these reasons, it is important to ensure that operations on signed integers do no result in overflow. (See MSC15-C. Do not depend on undefined behavior.) Of particular importance , however, are operations on signed integer values that originate from untrusted sources and are used in any of the following ways:
...
Code Block | ||||
---|---|---|---|---|
| ||||
signed int si1, si2, sum;
/* Initialize si1 and si2 */
if (((si2>0) && (si1 > (INT_MAX-si2)))
|| ((si2<0) && (si1 < (INT_MIN-si2)))) {
/* handle error condition */
}
else {
sum = si1 + si2;
}
|
This solution is more readable but may be less efficient than the solution that is specific to two's complement representation.
...
This noncompliant code example can result in a divide-by-zero error. Furthermore, many hardware platforms implement modulo as part of the division operator, which can overflow. Overflow can occur during a modulo operation when the dividend is equal to the minimum (negative) value for the signed integer type and the divisor is equal to−1to −1. This occurs despite that the result of such a modulo operation should theoretically be 0.
...
On MSVC++, taking the modulo of INT_MIN
by −1 yields the value 0. On gccGCC/Linux, taking the modulo of INT_MIN
by −1 produces a floating-point exception. However, on gcc on GCC versions 4.2.4 and newer, with optimization enabled, taking the modulo of INT_MIN
by −1 yields the value 0.
...
This compliant solution is based on the fact that both the division and modulo operators truncate toward zero, as specified in Section 6.5.5, footnote 105, of the C standard [ISO/IEC 9899:2011]. This guarantees that
Code Block |
---|
i % j |
...
This solution is also compliant with INT34-C. Do not shift a negative number of bits or more bits than exist in the operand.
Atomic Integers
The C Standard standard [ISO/IEC 9899:2011] defines the behavior of arithmetic on atomic signed integer types to use two's complement representation with silent wraparound on overflow; there are no undefined results. However, although defined, these results may be unexpected and therefore carry similar risks to unsigned integer wrapping (see INT30-C. Ensure that unsigned integer operations do not wrap). Consequently, signed integer overflow of atomic integer types should also be prevented or detected.
...
The CERT Oracle Secure Coding Standard for Java: INT00-J. Perform explicit range checking to avoid integer overflow
ISO/IEC 9899:2011 Section Section 6.5, "Expressions," and Section 7.10, "Sizes of integer types <limits.h>"
...
ISO/IEC TR 24772 "XYY Wrap-around Errorerror"
MITRE CWE: CWE-129, "Unchecked Array Indexingarray indexing"
MITRE CWE: CWE-190, "Integer Overflow overflow (Wrap wrap or Wraparoundwraparound)"
Bibliography
[Dowd 2006] Chapter 6, "C Language Issues" ("Arithmetic Boundary Conditionsboundary conditions," pp. 211–223)
[Seacord 2005] Chapter 5, "Integers"
[Viega 2005] Section 5.2.7, "Integer overflow"
[VU#551436]
[Warren 2002] Chapter 2, "Basics"
...