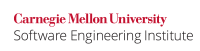
...
This example shows the use of the switch
statement to jump into a for
loop.:
Code Block | ||||
---|---|---|---|---|
| ||||
int f(int i) { int j=0; switch (i) { case 1: for(j=0;j<10;j++) { // No break, process case 2 as well case 2: // switch jumps inside the for block j++; // No break, process case 3 as well case 3: j++; } break; default: // Default action break; } return j; } |
...
The compliant example separates the switch
and for
blocks.:
Code Block | ||||
---|---|---|---|---|
| ||||
int f(int i) { int j=0; switch (i) { case 1: // No break, process case 2 as well case 2: j++; // No break, process case 3 as well case 3: j++; break; default: // Default action return j; } for(j++;j<10;j++) { j+=2; } return j; } |
...
Duff's device is a curious optimization applied to code intended to perform a serial copy. That is, it copies a series of bytes into one memory output in turn. A simple code to do this would be as follows:
Code Block |
---|
size_t count; /* Must be nonzero */ char *to; /* Output destination */ char *from; /* Points to count bytes to copy */ do { *to = *from++; /* Note that the "to" pointer is NOT incremented */ } while (--count > 0); |
...
This is an alternative implementation of Duff's device, which separates the switch
statement and loop.:
Code Block | ||||
---|---|---|---|---|
| ||||
int n = (count + 7) / 8; switch (count % 8) { case 0: *to = *from++; /* fall through */ case 7: *to = *from++; /* fall through */ case 6: *to = *from++; /* fall through */ case 5: *to = *from++; /* fall through */ case 4: *to = *from++; /* fall through */ case 3: *to = *from++; /* fall through */ case 2: *to = *from++; /* fall through */ case 1: *to = *from++; /* fall through */ } while (--n > 0) { *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; } |
...
CERT C++ Secure Coding Standard | MSC20-CPP. Do not use a switch statement to transfer control into a complex block |
ISO/IEC TR 24731-1:2007 | |
MISRA - C:2012 | Rule 15.116.2 (required) |
Bibliography
[ISO/IEC 9899:2011] | Section 6.8.6.1, "The goto Statement" |
Tom Duff on Duff's Device |
...