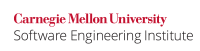
...
One way to inform the calling function of errors is to return a value indicating success or failure. This compliant solution ensures each function returns a value of type errno_t
, where 0 indicates that no error has occurred.:
Code Block | ||||
---|---|---|---|---|
| ||||
const errno_t ESOMETHINGREALLYBAD = 1; errno_t g(void) { /* ... */ if (something_really_bad_happens) { return ESOMETHINGREALLYBAD; } /* ... */ return 0; } errno_t f(void) { errno_t status = g(); if (status != 0) return status; /* ... do the rest of f ... */ return 0; } |
...
Instead of encoding status indicators in the return value, each function can take a pointer as an argument, which is used to indicate errors. In the following example, each function uses an errno_t\ *
argument to report errors.:
Code Block | ||||
---|---|---|---|---|
| ||||
const errno_t ESOMETHINGREALLYBAD = 1; void g(errno_t * err) { if (err == NULL) { /* Handle null pointer */ } /* ... */ if (something_really_bad_happens) { *err = ESOMETHINGREALLYBAD; } else { /* ... */ *err = 0; } } void f(errno_t * err) { if (err == NULL) { /* Handle null pointer */ } g(err); if (*err == 0) { /* ... do the rest of f ... */ } return 0; } |
...
Tool | Version | Checker | Description |
---|---|---|---|
Compass/ROSE |
|
| Could detect violations of this rule merely by reporting functions that call |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...
...