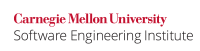
...
This compliant solution eliminates the possibility of str
, referencing nondynamic memory when it is supplied to free()
.:
Code Block | ||||
---|---|---|---|---|
| ||||
enum { MAX_ALLOCATION = 1000 }; int main(int argc, const char *argv[]) { char *str = NULL; size_t len; if (argc == 2) { len = strlen(argv[1])+1; if (len > MAX_ALLOCATION) { /* Handle error */ } str = (char *)malloc(len); if (str == NULL) { /* Handle allocation error */ } strcpy(str, argv[1]); } else { printf("%s\n", "usage: $>a.exe [string]"); return -1; } /* ... */ free(str); return 0; } |
...
In this noncompliant example, the pointer parameter to realloc()
, buf
, does not refer to dynamically allocated memory.:
Code Block | ||||
---|---|---|---|---|
| ||||
#define BUFSIZE 256 void f(void) { char buf[BUFSIZE]; char *p; /* ... */ p = (char *)realloc(buf, 2 * BUFSIZE); /* violation */ /* ... */ } |
...
In this compliant solution, buf
refers to dynamically allocated memory.:
Code Block | ||||
---|---|---|---|---|
| ||||
#define BUFSIZE 256 void f(void) { char *buf = (char *)malloc(BUFSIZE * sizeof(char)); char *p; /* ... */ p = (char *)realloc(buf, 2 * BUFSIZE); /* violation */ /* ... */ } |
...
MEM34-EX1: Some library implementations accept and ignore a deallocation of non-allocated nonallocated memory (or, alternatively, cause a runtime-constraint violation). If all libraries used by a project have been validated as having this behavior, then this rule can be ignored.
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Compass/ROSE | Can detect some violations of this rule. | ||||||||
| BAD_FREE | Identifies calls to | |||||||
| FNH.MIGHT | ||||||||
| 483 S | Fully implemented. |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...