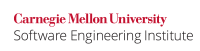
...
When a runtime-constraint violation is detected, the destination string is set to the null string (as long as it is not a null pointer, and the maximum length of the destination buffer is greater than 0 and not greater than RSIZE_MAX
), and the function returns a nonzero value. In the following example, the strcpy_s()
function is used to copy src1
to dst1
.:
Code Block |
---|
char src1[100] = "hello"; char src2[8] = {'g','o','o','d','b','y','e','\0'}; char dst1[6], dst2[5]; int r1, r2; r1 = strcpy_s(dst1, sizeof(dst1), src1); r2 = strcpy_s(dst2, sizeof(dst2), src2); |
...
The following noncompliant code overflows its buffer if msg
is too long, and it has undefined behavior if msg
is a null pointer.:
Code Block | ||||
---|---|---|---|---|
| ||||
void complain(const char *msg) { static const char prefix[] = "Error: "; static const char suffix[] = "\n"; char buf[BUFSIZ]; strcpy(buf, prefix); strcat(buf, msg); strcat(buf, suffix); fputs(buf, stderr); } |
...
The following compliant solution will not overflow its buffer.:
Code Block | ||||
---|---|---|---|---|
| ||||
void complain(const char *msg) { errno_t err; static const char prefix[] = "Error: "; static const char suffix[] = "\n"; char buf[BUFSIZ]; err = strcpy_s(buf, sizeof(buf), prefix); if (err != 0) { /* Handle error */ } err = strcat_s(buf, sizeof(buf), msg); if (err != 0) { /* Handle error */ } err = strcat_s(buf, sizeof(buf), suffix); if (err != 0) { /* Handle error */ } fputs(buf, stderr); } |
...