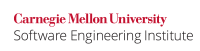
...
Noncompliant code example uses the identifier-list form for parameter declarations.:
Code Block | ||||
---|---|---|---|---|
| ||||
int max(a, b) int a, b; { return a > b ? a : b; } |
...
In this compliant solution, int
is the type specifier, max(int a, int b)
is the function declarator, and the block within the curly braces is the function body.:
Code Block | ||||
---|---|---|---|---|
| ||||
int max(int a, int b) { return a > b ? a : b; } |
...
Declaring a function without any prototype forces the compiler to assume that the correct number and type of parameters have been supplied to a function. This practice can result in unintended and undefined behavior.
In this noncompliant code example, the definition of func()
in file_a.c
expects three parameters but is supplied only two.:
Code Block | ||||
---|---|---|---|---|
| ||||
/* file_a.c source file */ int func(int one, int two, int three){ printf("%d %d %d", one, two, three); return 1; } |
However, because there is no prototype for func()
in file_b.c
, the compiler assumes that the correct number of arguments has been supplied and uses the next value on the program stack as the missing third argument.:
Code Block | ||||
---|---|---|---|---|
| ||||
/* file_b.c source file */ func(1, 2); |
...
This compliant solution correctly includes the function prototype for func()
in the compilation unit in which it is invoked, and the function invocation has been corrected to pass the right number of arguments.:
Code Block | ||||
---|---|---|---|---|
| ||||
/* file_b.c source file */ int func(int, int, int); func(1, 2, 3); |
...
To correct this example, the declaration of fn_ptr
is changed to accept three arguments.:
Code Block | ||||
---|---|---|---|---|
| ||||
int add(int x, int y, int z) { return x + y + z; } int main(int argc, char *argv[]) { int (*fn_ptr) (int, int, int) ; int res; fn_ptr = add; res = fn_ptr(2, 3, 4); /* ... */ return 0; } |
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
| decltype | Fully implemented. | |||||||
GCC |
|
| Can detect violation of this recommendation when the | ||||||
| 21 S | Fully implemented. | |||||||
PRQA QA-C |
| 3335 | Fully implemented. |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
...
ISO/IEC TR 24772:2013 | Type System [IHN] Subprogram Signature Mismatch [OTR] |
MISRA - C:2012 | Rule 8.21 (required) |
Bibliography
[ISO/IEC 9899:2011] | Section 6.11.7, "Function Definitions" |
[Spinellis 2006] | Section 2.6.1, "Incorrect Routine or Arguments" |
...