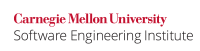
Bit-fields can be used to allow flags or other integer values with small ranges to be packed together to save storage space.
For bit-fields, it It is implementation-defined whether the specifier int
designates the same type as signed int
or the same type as unsigned int
for bit-fields. Also, C99 also requires that "If an int
can represent all values of the original type, the value is converted to an int
; otherwise, it is converted to an unsigned int
."
...
Code Block |
---|
struct { unsigned int a: 8; } bits = {255}; int main(void) { printf("unsigned 8-bit field promotes to %s.\n", (bits.a <<- 24)256 <> 0) ? "signed" : "unsigned"); } |
The type of the expression (bits.a << 24- 256 > 0)
is compiler dependent and may be either signed or unsigned depending on the compiler implementor's interpretation of the standard.
...
The second interpretation is that (bits.a
is an 8-bit integer. As a result, this eight bit value can be represented as an int
, so integer promotions require that it be converted to int
, and hence "signed". In the signed case, the 24-bit left shift tries to compute 0xFF000000
. For implementations where int is only 32 bits wide, 0xFF000000 > INT_MAX
and the overflow produces undefined behavior (See C99 Sections 3.4.3p3 (non-normative example), 6.5p5 (normative), and 6.5.7p4 (normative)).
The type of the bitfield bit-field when used in an expression also has implications for signed long long
and unsigned long long
types. Compilers that determine the size of the
For example, gcc interprets the following as an eight bit value and promote it to int
:
...
The following attributes of bit-fields are also implemention implementation defined:
- The alignment of bit fields in the storage unit. For example, the bit fields may be allocated from the high end or the low end of the storage unit.
- Whether or not bit-fields can overlap an storage unit boundary. For example, assuming eight bits to a byte, if bit-fields of six and four bits are declared, is each bitfield contained within a byte or are they be split across multiple bytes?
...