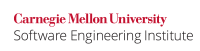
...
Declaring both length
and the blocksize
argument to alloc()
as rsize_t
eliminates the possibility of truncation. This compliant solution assumes that read_integer_from_network()
and read_network_data()
can also be modified to accept a length argument of type pointer to rsize_t
and rsize_t
, respectively. If these functions are part of an external library that cannot be updated, care must be taken when casting length
into an unsigned long
to unsure that no overflow occurs.
Code Block | ||
---|---|---|
| ||
void *alloc(rsize_t blocksize) { if (blocksize > RSIZE_MAX) { /* Handle error */ } return malloc(blocksize); } int read_counted_string(int fd) { rsize_t length; unsigned char *data; if (read_integer_from_network(fd, &length) < 0) { return -1; } if (length >= RSIZE_MAX) { /* handle integer overflow */ } data = (unsigned char*)alloc(length + 1); if (data == NULL) { /* Handle Error */ } if (read_network_data(fd, data, length) < 0) { free(data); return -1; } data[length] = '\0'; /* ... */ free( data); return 0; } |
...