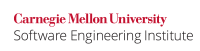
Macros are often used to generalize several segments of code which might be used multiple times in the code. This guideline focuses on macros consisting of single statements, which do not usually have to be enclosed in a do()....
-while()
. (See also PRE10-C. Wrap multi-statement macros in a do-while loop.)
...
Code Block | ||
---|---|---|
| ||
#define FOR_LOOP(n) for(i=0; i<(n); i++); main() { int i; FOR_LOOP(3) { printfputs("Inside for loop\n"); } } |
The user expects to get the following output from the code:
...
Code Block | ||
---|---|---|
| ||
#define FOR_LOOP(n) for(i=0; i<(n); i++) main() { int i; FOR_LOOP(3) { printfputs("Inside for loop\n"); } } |
Noncompliant Code Example
In the this noncompliant code belowexample, the programmer defines a macro which increments the value in the argument 1 by one and modulates it with the max value provided by the user.
Code Block | ||
---|---|---|
| ||
#define INCREMENT(x, max) ((x) = ((x) + 1) % (max)); main() { int index = 0; int value; value = INCREMENT(index, 10) + 2; /* ........... ........... } */ |
In this caseIn the above code, the programmer intends to increment index
and then use that as a value by adding 2 to it. Unfortunately, the value will always be is equal to the incremented value of index
because of the semicolon present at the end of the macro. The '+ 2;'
will be is treated as another a separate statement by the compiler. The user will not get any compilation errors. If the user has not enabled warnings while compiling, the effect of the semicolon in the macro cannot be detected at an early stage.
...
Code Block | ||
---|---|---|
| ||
#define INCREMENT(x, max) ((x) = ((x) + 1) % (max)) main() { int index = 0; int value; value = INCREMENT(index, 10) + 2; ......... .......... } |
Mitigation Strategies
The programmer should ensure that there is no semicolon at the end of a macro definition with single statement. The responsibility for having a semicolon where needed during the use of the macro should be given to the person using the macro.
...