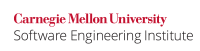
Do not use the assignment operator in the following cases because it typically indicates programmer error and can result in unexpected behavior:
- Controlling expression of
if
,switch
(selection statement) - Controlling expression of
while
,do...while
(iteration statement) - S
econd operand of for
(iteration statement) - First operand of
?:
(selection statement) - Either operand of
||
or&&
(logical operators) - Second operand of comma operator when the comma expression is used in any of these contexts
- Second and third operands of of
?:
(selection statement) where the ternary expression is used in any of these contexts
...
Although the intent of the code may be to assign b
to a
and test the value of the result for equality to zero0, it is very frequently a case of the programmer mistakenly using the assignment operator =
instead of the equals operator ==
. Consequently, many compilers will warn about this condition, making this coding error detectable by adhering to MSC00-C. Compile cleanly at high warning levels.
...
Code Block | ||||
---|---|---|---|---|
| ||||
do { /* ... */ } while ( foo(), (x = y) != 0) ; |
Noncompliant Code Example
In this noncompliant example, the expression p = q
is used as the controlling expression of the while
statement:
Code Block | ||||
---|---|---|---|---|
| ||||
do { /* ... */ } while ( x = y, p = q ) ; |
Compliant Solution
This is a compliant example because the expression x = y
is not used as the controlling expression of the while
statement:
Code Block | ||||
---|---|---|---|---|
| ||||
do { /* ... */ } while ( x = y, p == q ) ; |
Exceptions
EXP18-EX1: Assignment can be used where the result of the assignment is itself a parameter to a comparison expression or relational expression. In this compliant example, the expression x = y
is itself a parameter to a comparison operation:
...
EXP18-EX2: Assignment can be used where the expression consists of a single primary expression. In this compliant example, the expression x = y
is a single primary expression:
...
EXP18-EX3: Assignment can be used in the above contexts if it occurs in a function argument or array index. In this compliant example, the expression x = y
is used in a function argument:
...
Code Block | ||||
---|---|---|---|---|
| ||||
if ( foo( x = y ) ) { /* ... */ } |
This is a noncompliant example because &&
is not a comparison or relational operator and the entire expression is not primary:
Code Block | ||||
---|---|---|---|---|
| ||||
if ( ( v = w ) && flag ) { /* ... */ } |
When the assignment of v
to w
is not intended, this conditional block is now executed when v
is equal to w
:
Code Block | ||||
---|---|---|---|---|
| ||||
if ( ( v == w ) && flag ) { /* ... */ }; |
When the assignment is intended, the following is an alternative compliant solution:
Code Block | ||||
---|---|---|---|---|
| ||||
if ( ( (v = w) != 0 ) && flag ) { /* ... */ }; |
Risk Assessment
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
|
| Could detect violations of this recommendation by identifying any assignment expression as the top-level expression in an | |||||||
| CC2.EXP18 | Fully implemented | |||||||
|
| Can detect violations of this recommendation when the | |||||||
| ASSIGCOND.GEN |
| |||||||
| 9 S |
| |||||||
PRQA QA-C |
| 3314 | Partially implemented |
...