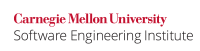
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> /* For malloc() and size_t */ struct flexArrayStruct { int num; int data[1]; }; /* ... */ size_t array_size; size_t i; void func(void) { /* Initialize array_size */ /* Space is allocated for the struct */ struct flexArrayStruct *structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * (array_size - 1)); if (structP == NULL) { /* Handle malloc failure */ } structP->num = 0; /* Access data[] as if it had been allocated * as data[array_size] */ for (i = 0; i < array_size; i++) { structP->data[i] = 1; } } |
The problem with using this approach is that the behavior is undefined when accessing other than the first element of data. (See subclause 6.5.6 of the C Standard [ISO/IEC 9899:2011].) Consequently, the compiler can generate code that does not return the expected value when accessing the second element of data.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> /* For malloc() and size_t */ struct flexArrayStruct{ int num; int data[]; }; /* ... */ size_t array_size; size_t i; void func(void) { /* Initialize array_size */ /* Space is allocated for the struct */ struct flexArrayStruct *structP = (struct flexArrayStruct *) malloc(sizeof(struct flexArrayStruct) + sizeof(int) * array_size); if (structP == NULL) { /* Handle malloc failure */ } structP->num = 0; /* Access data[] as if it had been allocated * as data[array_size] */ for (i = 0; i < array_size; i++) { structP->data[i] = 1; } } |
This compliant solution allows the structure to be treated as if it had declared the member data[]
to be data[array_size]
in a manner that conforms to the C Standard. Note that Microsoft Visual Studio implements support for flexible array members, but some versions (such as MSVC 11) warn that this is a nonstandard extension.
...