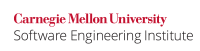
Subclause 6.2.5, paragraph 9, of the C Standard [ISO/IEC 9899:2011] states:
A computation involving unsigned operands can never overflow, because a result that cannot be represented by the resulting unsigned integer type is reduced modulo the number that is one greater than the largest value that can be represented by the resulting type.
...
Although unsigned left shift <<
can result in wrapping, modulo behavior is permitted by this standard because of common usage, because this behavior is usually expected by the programmer , and because the behavior is well - defined.
The following sections examine specific operations that are susceptible to unsigned integer wrap. When operating on small integer types (smaller than int
), integer promotions are applied. The usual arithmetic conversions may also be applied to (implicitly) convert operands to equivalent types before arithmetic operations are performed. Make sure you understand integer conversion rules before trying to implement secure arithmetic operations. (See INT02-C. Understand integer conversion rules.)
...
This noncompliant code example may result in an unsigned integer wrap during the addition of the unsigned operands ui_a
and ui_b
. If this behavior is unexpected, the resulting value may be used to allocate insufficient memory for a subsequent operation or in some other manner that can lead to an exploitable vulnerability.
...
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned int ui_a; unsigned int ui_b; unsigned int usum; /* Initialize ui_a and ui_b */ if (UINT_MAX - ui_a < ui_b) { /* handleHandle error condition */ } else { usum = ui_a + ui_b; } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned int ui_a; unsigned int ui_b; unsigned int usum; /* Initialize ui_a and ui_b */ usum = ui_a + ui_b; if (usum < ui_a) { /* handleHandle error condition */ } |
Anchor | ||||
---|---|---|---|---|
|
...
Subtraction is between two operands of arithmetic type, between two pointers to qualified or unqualified versions of compatible object types, or between a pointer to an object type and an integer type. (See ARR36-C. Do not subtract or compare two pointers that do not refer to the same array, ARR37-C. Do not add or subtract an integer to a pointer to a non-array object, and ARR38-C. Do not add or subtract an integer to a pointer if the resulting value does not refer to a valid array element for information about pointer subtraction.) Decrementing is equivalent to subtracting 1.
...
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned int ui_a; unsigned int ui_b; unsigned int udiff; /* Initialize ui_a and ui_b */ if (ui_a < ui_b){ /* handleHandle error condition */ } else { udiff = ui_a - ui_b; } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
unsigned int ui_a; unsigned int ui_b; unsigned int udiff; /* Initialize ui_a and ui_b */ udiff = ui_a - ui_b; if (udiff > ui_a) { /* handleHandle error condition */ } |
Anchor | ||||
---|---|---|---|---|
|
...
Code Block | ||||
---|---|---|---|---|
| ||||
pen->num_vertices = _cairo_pen_vertices_needed( gstate->tolerance, radius, &gstate->ctm ); if (pen->num_vertices > SIZE_MAX/sizeof(cairo_pen_vertex_t)) { /* handleHandle error condition */ } pen->vertices = malloc( pen->num_vertices * sizeof(cairo_pen_vertex_t) ); |
...
Code Block | ||||
---|---|---|---|---|
| ||||
atomic_int i; int ui_a; /* Initialize ui_a, i */ atomic_fetch_add(&i, ui_a); if (atomic_load(&i) < ui_a) { /* handleHandle error condition */ } |
Exceptions
...