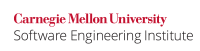
...
The following noncompliant code determines the square root of x
.
Code Block | ||||
---|---|---|---|---|
| ||||
double x; double result; result = sqrt(x); |
...
Since this function has domain errors but no range errors, one can use bounds checking to prevent domain errors.
Code Block | ||||
---|---|---|---|---|
| ||||
double x; double result; if (isless(x, 0)) { /* handle domain error */ } result = sqrt(x); |
...
This noncompliant code example determines the hyperbolic cosine of x
.
Code Block | ||||
---|---|---|---|---|
| ||||
double x; double result; result = cosh(x); |
...
Since this function has no domain errors but may have range errors, one must detect a range error and act accordingly.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; double x; double result; result = sinh(x); #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* handle range error */ } #endif |
...
The following noncompliant code raises x
to the power of y
.
Code Block | ||||
---|---|---|---|---|
| ||||
double x; double y; double result; result = pow(x, y); |
...
Since the pow()
function can produce both domain errors and range errors, we must first check that x
and y
lie within the proper domain. We must also detect if a range error occurs, and act accordingly.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; /* call the function */ double x; double y; double result; if (((x == 0.f) && islessequal(y, 0)) || (isless(x, 0))) { /* handle domain error */ } result = pow(x, y); #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* handle range error */ } #endif |
...