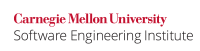
...
A small collection of macros can provide secure implementations for common uses for the standard memory allocation functions. The omission of a REALLOC()
macro is intentional . (See void MEM08see EXP39-C. Use realloc() only to resize dynamically allocated arrays.)Do not access a variable through a pointer of an incompatible type).
Code Block | ||||
---|---|---|---|---|
| ||||
/* Allocates a single object using malloc() */ #define MALLOC(type) ((type *)malloc(sizeof(type))) /* Allocates an array of objects using malloc() */ #define MALLOC_ARRAY(number, type) \ ((type *)malloc((number) * sizeof(type))) /* * Allocates a single object with a flexible * array member using malloc(). */ #define MALLOC_FLEX(stype, number, etype) \ ((stype *)malloc(sizeof(stype) \ + (number) * sizeof(etype))) /* Allocates an array of objects using calloc() */ #define CALLOC(number, type) \ ((type *)calloc(number, sizeof(type))) /* Reallocates an array of objects using realloc() */ #define REALLOC_ARRAY(pointer, number, type) \ ((type *)realloc(pointer, (number) * sizeof(type))) /* * Reallocates a single object with a flexible * array member using realloc(). */ #define REALLOC_FLEX(pointer, stype, number, etype) \ ((stype *)realloc(pointer, sizeof(stype) \ + (number) * sizeof(etype))) |
...
If one or more of the operands to the multiplication operations used in many of these macro definitions can be influenced by untrusted data, these operands should be checked for overflow before the macro is invoked . (See see INT32-C. Ensure that operations on signed integers do not result in overflow).)
The use of type-generic function-like macros is an allowed exception (PRE00-EX4) to PRE00-C. Prefer inline or static functions to function-like macros.
...
MEM02-EX1: Do not immediately cast the results of malloc()
for code that will be compiled using a C90-conforming compiler because it is possible for the cast to hide a more critical defect . See (see DCL31-C. Declare identifiers before using them for a code example that uses malloc() without without first declaring it).
Risk Assessment
Failing to cast the result of a memory allocation function call into a pointer to the allocated type can result in inadvertent pointer conversions. Code that follows this recommendation will compile and execute equally well in C++.
...