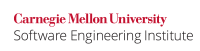
...
This noncompliant code example accesses the envp
pointer after calling setenv()
.
Code Block | ||||
---|---|---|---|---|
| ||||
int main(int argc, const char *argv[], const char *envp[]) { size_t i; if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle error */ } if (envp != NULL) { for (i = 0; envp[i] != NULL; i++) { if (puts(envp[i]) == EOF) { /* Handle error */ } } } return 0; } |
...
Use environ
in place of envp
when defined.
Code Block | ||||
---|---|---|---|---|
| ||||
extern char **environ; /* ... */ int main(int argc, const char *argv[]) { size_t i; if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle error */ } if (environ != NULL) { for (i = 0; environ[i] != NULL; i++) { if (puts(environ[i]) == EOF) { /* Handle error */ } } } return 0; } |
...
This noncompliant code example accesses the envp
pointer after calling _putenvs()
.
Code Block | ||||
---|---|---|---|---|
| ||||
int main(int argc, const char *argv[], const char *envp[]) { size_t i; if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle error */ } if (envp != NULL) { for (i = 0; envp[i] != NULL; i++) { if (puts(envp[i]) == EOF) { /* Handle error */ } } } return 0; } |
...
Use _environ
in place of envp
when defined.
Code Block | ||||
---|---|---|---|---|
| ||||
_CRTIMP extern char **_environ; /* ... */ int main(int argc, const char *argv[]) { size_t i; if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle error */ } if (_environ != NULL) { for (i = 0; _environ[i] != NULL; i++) { if (puts(_environ[i]) == EOF) { /* Handle error */ } } } return 0; } |
...
with
Code Block | ||||
---|---|---|---|---|
| ||||
#if defined (_POSIX_) || defined (__USE_POSIX) extern char **environ; #define envp environ #else _CRTIMP extern char **_environ; #define envp _environ #endif int main(int argc, char *argv[]) { /* ... */ } |
...