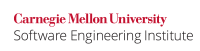
...
In this noncompliant code example, the signal handler handler()
is bound to signum
.
Code Block | ||||
---|---|---|---|---|
| ||||
void handler(int signum) { if (signal(signum, handler) == SIG_ERR) { /* Handle error */ } /* Handle signal */ } /* ... */ if (signal(SIGUSR1, handler) == SIG_ERR) { /* Handle error */ } |
...
For persistent platforms, calling the signal()
function from within the signal handler is unnecessary.
Code Block | ||||
---|---|---|---|---|
| ||||
void handler(int signum) { /* Handle signal */ } /* ... */ if (signal(SIGUSR1, handler) == SIG_ERR) { /* Handle error */ } |
...
POSIX defines the sigaction()
function, which assigns handlers to signals like signal()
but also allows the caller to explicitly set persistence. Consequently, the sigaction()
function can be used to eliminate the race window on nonpersistent operating systems.
Code Block | ||||
---|---|---|---|---|
| ||||
void handler(int signum) { /* Handle signal */ } /* ... */ struct sigaction act; act.sa_handler = handler; act.sa_flags = 0; if (sigemptyset( &act.sa_mask) != 0) { /* Handle error */ } if (sigaction(SIGUSR1, &act, NULL) != 0) { /* Handle error */ } |
...
The following code example resets a signal handler to the system's default behavior.
Code Block | ||||
---|---|---|---|---|
| ||||
void handler(int signum) { #ifndef WINDOWS if (signal(signum, SIG_DFL) == SIG_ERR) { /* Handle error */ } #endif /* Handle signal */ } /* ... */ if (signal(SIGUSR1, handler) == SIG_ERR) { /* Handle error */ } |
...