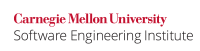
...
It is not necessary to go beyond the standard C library to find examples that violate this recommendation because the C language often prioritizes performance at the expense of robustness. The following are two examples from C99 §7.21.
Code Block | ||||
---|---|---|---|---|
| ||||
char *strncpy(char * restrict s1, const char * restrict s2, size_t n); char *strncat(char * restrict s1, const char * restrict s2, size_t n); |
...
The C99 strncpy()
and strncat()
functions could be improved by adding element count parameters as follows:
Code Block | ||||
---|---|---|---|---|
| ||||
char *strncpy(char * restrict s1, size_t s1count, const char * restrict s2, size_t s2count, size_t n); char *strncat(char * restrict s1, size_t s1count, const char * restrict s2, size_t s2count, size_t n); |
...
TR 24731-1, which will be an appendix in C1X, defines bounds-checking versions of standard C library string handling functions.
Code Block | ||||
---|---|---|---|---|
| ||||
errno_t strncpy_s(char * restrict s1, rsize_t s1max, const char * restrict s2, rsize_t n); errno_t strcat_s(char * restrict s1, rsize_t s1max, const char * restrict s2); |
...
TR 24731-1, which will be an appendix in C1X, defines bounds-checking versions of standard C library string handling functions.
Code Block | ||||
---|---|---|---|---|
| ||||
errno_t strcat_s(char * restrict s1, rsize_t s1max, const char * restrict s2); |
As another example, consider strcpy_s()
:
Code Block | ||||
---|---|---|---|---|
| ||||
errno_t strcpy_s(char * restrict s1, rsize_t s1max, const char * restrict s2); |
...