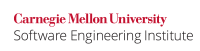
...
This example shows the use of the switch statement to jump into a for loop.
Code Block | ||||
---|---|---|---|---|
| ||||
int f(int i) { int j=0; switch (i) { case 1: for(j=0;j<10;j++) { // no break, process case 2 as well case 2: // switch jumps inside the for block j++; // no break, process case 3 as well case 3: j++; } break; default: // default action break; } return j; } |
...
The compliant example separates the switch and for blocks.
Code Block | ||||
---|---|---|---|---|
| ||||
int f(int i) { int j=0; switch (i) { case 1: // no break, process case 2 as well case 2: j++; // no break, process case 3 as well case 3: j++; break; default: // default action return j; } for(j++;j<10;j++) { j+=2; } return j; } |
...
However, this code might be unacceptably slow because the while condition is performed count
times. The classic code for Duff's Device unrolls this loop to minimize the number of comparisons performed:
Code Block | ||||
---|---|---|---|---|
| ||||
int n = (count + 7) / 8; switch (count % 8) { case 0: do { *to = *from++; case 7: *to = *from++; case 6: *to = *from++; case 5: *to = *from++; case 4: *to = *from++; case 3: *to = *from++; case 2: *to = *from++; case 1: *to = *from++; } while (--n > 0); } |
...
This is an alternate implemention of Duff's Device which separates the switch statement and loop.
Code Block | ||||
---|---|---|---|---|
| ||||
int n = (count + 7) / 8; switch (count % 8) { case 0: *to = *from++; /* fall through */ case 7: *to = *from++; /* fall through */ case 6: *to = *from++; /* fall through */ case 5: *to = *from++; /* fall through */ case 4: *to = *from++; /* fall through */ case 3: *to = *from++; /* fall through */ case 2: *to = *from++; /* fall through */ case 1: *to = *from++; /* fall through */ } while (--n > 0) { *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; *to = *from++; } |
...