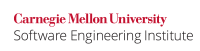
...
Code Block |
---|
main(int argc, char **argv, char **envp) \{\} |
Wiki Markup |
---|
According to |
C99 \[[ISO/IEC 9899-1999:TC2|AA. C References#ISO/IEC 9899-1999TC2]\]: |
In a hosted environment, the main function receives a third argument, char *envp[], that points to a null-terminated array of pointers to char, each of which points to a string that provides information about the environment for this execution of the program.
...
Non-Compliant Coding Example
After a call to setenv()
, environment pointers to the old value and copies of the old value may be incorrect envp
may not have the proper environment values.
Code Block | ||
---|---|---|
| ||
int main(int argc, char *temp; *argv, char *copy; if ((temp = getenv("TEST_ENV")) != NULL) { copy = malloc(strlen(temp) + 1); if (copy*envp) { setenv("MY_NEW_VAR", "/usr/bin", 1); if (envp != NULL) { strcpy(copy, temp); } else { /* handle error condition */ } } /* ...program code... */ setenv("TEST_ENV", var, 1); /* ...program code... */ printf("TEST_ENV: %s\n", temp); printf("TEST_ENV: %s\n", copy); |
Neither of the print statements will be correct.
Compliant Solution
for (i = 0; envp[i] != NULL; i++) {
printf("%s\n", envp[i]);
}
}
return 0;
}
|
MY_NEW_VAR
will not be found in envp
.
Compliant Solution (POSIX)
Use environ
in place of envp
You should always fetch fresh copies of environment variables, especially if you know that a value has changed.
Code Block | ||
---|---|---|
| ||
extern char *temp; char *copyenviron; ifint main((tempint = getenv("TEST_ENV")) != NULLargc, char **argv) { copy = malloc(strlen(temp) +setenv("MY_NEW_VAR", "/usr/bin", 1); if (copyenviron != NULL) { for (i strcpy(copy, temp); } else= 0; environ[i] != NULL; i++) { /* handle error condition */ } } /* ...program code... */ setenv("TEST_ENV", var, 1); /* ...program code... */ if ((temp = getenv("TEST_ENV")) != NULL) { copy = malloc(strlen(temp) + 1); if (copy != NULL) { strcpy(copy, temp); } else { /* handle error condition */ } } printf("TEST_ENV: %s\n", temp); printf("TEST_ENV: %s\n", copy); |
...
printf("%s\n", environ[i]);
}
}
return 0;
}
|
Note: if you have a great deal of unsafe envp
code, you could save time in your remediation by aliasing. Change:
Code Block |
---|
main(int argc, char **argv, char **envp)
|
To:
Code Block |
---|
extern char **environ;
#define envp environ
main(int argc, char **argv)
|
Risk Assessment
The program would not be using current environment values, causing unexpected results.
...