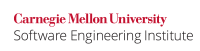
...
Code Block | ||
---|---|---|
| ||
void strtr(char *str, char orig, char rep) { while (*str != '\0') { if (*str == orig) { *str = rep; } str++; } } /* ... */ char *env = getenv("TEST_ENV"); if (env == NULL) { /* Handle Errorerror */ } strtr(env,'"', '_'); /* ... */ |
...
For the case where the intent of the noncompliant code example is to use the modified value of the environment variable locally and not modify the environment, this compliant solution makes a local copy of that string value , and then modifies the local copy.
Code Block | ||
---|---|---|
| ||
const char *env; char *copy_of_env; env = getenv("TEST_ENV"); if (env == NULL) { /* Handle Errorerror */ } copy_of_env = (char *)malloc(strlen(env) + 1); if (copy_of_env == NULL) { /* Handle Errorerror */ } strcpy(copy_of_env, env); strtr(copy_of_env,'\"', '_'); |
Compliant Solution (
...
Modifying the
...
Environment in POSIX)
For the case where the intent is to modify the environment, this compliant solution will save saves the altered string back into the environment by using the POSIX setenv()
and strdup()
functions.
Code Block | ||
---|---|---|
| ||
const char *env; char *copy_of_env; env = getenv("TEST_ENV"); if (env == NULL) { /* Handle Errorerror */ } copy_of_env = strdup(env); if (copy_of_env == NULL) { /* Handle Errorerror */ } strtr(copy_of_env,'\"', '_'); if (setenv("TEST_ENV", copy_of_env, 1) != 0) { /* Handle Errorerror */ } |
Risk Assessment
The modified string may be overwritten by a subsequent call to the getenv()
function. Depending on the implementation, modifying the string returned by getenv()
may or may not modify the environment.
...