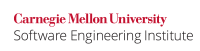
...
The argument to malloc()
can be any value of (unsigned) type size_t
. If the program uses the allocated storage to represent an object (possibly an array) whose size is greater than the requested size, the behavior is undefined. The implicit pointer conversion lets this slip by without complaint from the compiler.
For Consider the following example:
Code Block | ||
---|---|---|
| ||
#include <stdlib.h> typedef struct gadget gadget; struct gadget { int i; double d; }; typedef struct widget widget; struct widget { char c[10]; int i; double d; }; widget *p; /* ... */ p = malloc(sizeof(gadget)); /* imminent problem */ if (p != NULL) { p->i = 0; /* undefined behavior */ p->d = 0.0; /* undefined behavior */ } |
...
This lets the compiler detect the invalid assignment , because it attempts to convert a gadget *
into a widget *
.
...
Code Block | ||
---|---|---|
| ||
widget *p; /* ... */ p = (widget *)malloc(sizeof(widget)); |
Compliant Solution (
...
Macros)
Repeating the same type in the sizeof
expression and the pointer cast is easy to do but still invites errors. Packaging the repetition in a macro, such as
...
A small collection of macros can provide secure implementations for common uses for the standard memory allocation functions. The omission of a REALLOC()
macro is intentional. (see See guideline MEM08-C. Use realloc() only to resize dynamically allocated arrays.).
Code Block | ||
---|---|---|
| ||
/* allocates a single object using malloc(). */ #define MALLOC(type) ((type *)malloc(sizeof(type))) /* allocates an array of objects using malloc(). */ #define MALLOC_ARRAY(number, type) \ ((type *)malloc((number) * sizeof(type))) /* allocates a single object with a flexible * array member using malloc(). */ #define MALLOC_FLEX(stype, number, etype) \ ((stype *)malloc(sizeof(stype) \ + (number) * sizeof(etype))) /* allocates an array of objects using calloc(). */ #define CALLOC(number, type) \ ((type *)calloc(number, sizeof(type))) /* reallocates an array of objects using realloc(). */ #define REALLOC_ARRAY(pointer, number, type) \ ((type *)realloc(pointer, (number) * sizeof(type))) /* reallocates a single object with a flexible * array member using realloc(). */ #define REALLOC_FLEX(pointer, stype, number, etype) \ ((stype *)realloc(pointer, sizeof(stype) \ + (number) * sizeof(etype))) |
For The following is an example:
Code Block | ||
---|---|---|
| ||
enum month { Jan, Feb, /* ... */ }; typedef enum month month; typedef struct date date; struct date { unsigned char dd; month mm; unsigned yy; }; typedef struct string string; struct string { size_t length; char text[]; }; date *d, *week, *fortnight; string *name; d = MALLOC(date); week = MALLOC_ARRAY(7, date); name = MALLOC_FLEX(string, 16, char); fortnight = CALLOC(14, date); |
If one or more of the operands to the multiplication operations used in many of these macro definitions can be influenced by untrusted data, these operands should be checked for overflow before invoking the macro. (see See guideline INT32-C. Ensure that operations on signed integers do not result in overflow.).
The use of type-generic function-like macros is an allowed exception (PRE00-EX4) to guideline PRE00-C. Prefer inline or static functions to function-like macros.
...
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM02-C | low | unlikely | low | P3 | L3 |
Automated Detection
...
Tool | Version | Checker | Description |
---|---|---|---|
|
...
|
|
| ||||||||
|
|
|
|
...
|
...
|
|
|
|
...
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
Related Guidelines
This rule appears in the C++ Secure Coding Standard as : MEM02-CPP. Immediately cast the result of a memory allocation function call into a pointer to the allocated type.
Bibliography
Wiki Markup |
---|
\[[ISO/IEC 9899:1999|AA. Bibliography#ISO/IEC 9899-1999]\] Section 7.20.3, "Memory management functions" \[[Summit 052005|AA. Bibliography#Summit 05]\] [Question 7.7|http://c-faq.com/malloc/cast.html] and [Question 7.7b|http://c-faq.com/malloc/mallocnocast.html] |
...