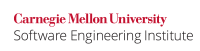
...
Wiki Markup |
---|
The exact treatment of error conditions from math functions is quite complicated. C99 Section 7.12.1 defines the following behavior for floating point overflow \[[ISO/IEC 9899:1999|AA. Bibliography#ISO/IEC 9899-1999]\]: |
A floating result overflows if the magnitude of the mathematical result is finite but so large that the mathematical result cannot be represented without extraordinary roundoff error in an object of the specified type. If a floating result overflows and default rounding is in effect, or if the mathematical result is an exact infinity from finite arguments (for example
log(0.0)
), then the function returns the value of the macroHUGE_VAL
,HUGE_VALF
, orHUGE_VALL
according to the return type, with the same sign as the correct value of the function; if the integer expressionmath_errhandling & MATH_ERRNO
is nonzero, the integer expressionerrno
acquires the valueERANGE
; if the integer expressionmath_errhandling & MATH_ERREXCEPT
is nonzero, the ''divide-by-zero'' floating-point exception is raised if the mathematical result is an exact infinity and the ''overflow'' floating-point exception is raised otherwise.
...
Wiki Markup |
---|
It is also difficult to check for math errors using {{errno}} because an implementation might not set it. For real functions, the programmer can tell whether the implementation sets {{errno}} by checking whether {{math_errhandling & MATH_ERRNO}} is nonzero. For complex functions, the C99, Section 7.3.2, simply states "an implementation may set {{errno}} but is not required to" \[[ISO/IEC 9899:1999|AA. Bibliography#ISO/IEC 9899-1999]\]. |
...
Code Block |
---|
#include <math.h> #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) #include <fenv.h> #endif /* ... */ #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) feclearexcept(FE_ALL_EXCEPT); #endif errno = 0; /* call the function */ #if !defined(math_errhandling) \ || (math_errhandling & MATH_ERRNO) if (errno != 0) { /* handle range error */ } #endif #if defined(math_errhandling) \ && (math_errhandling & MATH_ERREXCEPT) if (fetestexcept(FE_INVALID | FE_DIVBYZERO | FE_OVERFLOW | FE_UNDERFLOW) != 0) { /* handle range error */ } #endif |
See guideline FLP03-C. Detect and handle floating point errors for more details on how to detect floating point errors.
...
The following noncompliant code determines the square root of x
.
Code Block | ||
---|---|---|
| ||
double x; double result; result = sqrt(x); |
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
FLP32-C | medium | probable | medium | P8 | L2 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
|
...
|
...
|
|
...
|
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
Related Guidelines
This rule appears in the C++ Secure Coding Standard as : FLP32-CPP. Prevent or detect domain and range errors in math functions.
Bibliography
Wiki Markup |
---|
\[[ISO/IEC 9899:1999|AA. Bibliography#ISO/IEC 9899-1999]\] Section 7.3, "Complex arithmetic <{{complex.h}}>", and Section 7.12, "Mathematics <{{math.h}}>" \[[MITRE 072007|AA. Bibliography#MITRE 07]\] [CWE ID 682|http://cwe.mitre.org/data/definitions/682.html], "Incorrect Calculation" \[[Plum 851985|AA. Bibliography#Plum 85]\] Rule 2-2 \[[Plum 891989|AA. Bibliography#Plum 91]\] Topic 2.10, "conv - conversions and overflow" |
...