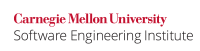
...
In general, do not use scanf()
to parse integers or floating-point numbers from input strings, because the input could contain numbers not representable by the argument type.
Compliant Solution (Linux)
This compliant example uses the Linux scanf()
implementation's built in error handling to validate input. On Linux platforms, scanf()
sets errno
to ERANGE
if the result of integer conversion cannot be represented within the size specified by the format string. Note that this is a platform dependent solution. Therefore, this should only be used where portability is not a concern.
Code Block | ||
---|---|---|
| ||
long sl;
errno = 0;
if (scanf("%ld", &sl) != 1) {
/* handle error */
}
else if (ERANGE == errno) {
if (puts("number out of range\n") == EOF) {
/* Handle error */
}
}
|
Compliant Solution
This compliant example uses fgets()
to input a string and strtol()
to convert the string to an integer. Error checking is provided to make sure that the value is a valid integer in the range of long
.
...
Note that this solution treats any trailing characters, including white-space characters, as an error condition.
Compliant Solution
This compliant example uses the Linux scanf()
implementation's built in error handling to validate input. On Linux platforms, scanf()
sets errno
to ERANGE
if integer conversion would cause overflow with the given type. Note that this is a platform dependent solution. Therefore, this should only be used where portability is not a concern.
...
bgColor | #ccccff |
---|
...
Risk Assessment
While it is relatively rare for a violation of this recommendation to result in a security vulnerability, it can easily result in lost or misinterpreted data.
...