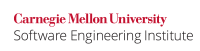
...
Addition is between two operands of arithmetic type or between a pointer to an object type and an integer type . Incrementing is equivalent to adding one.
Non-Compliant Code Example
This code may result in a signed integer overflow during the addition of the signed operands si1
and si2
. If this behavior is unanticipated, it could lead to an exploitable vulnerability.
(see ARR37-C. Do not add or subtract an integer to a pointer to a non-array object and ARR38-C. Do not add or subtract an integer to a pointer if the resulting value does not refer to an element within the array for rules about adding a pointer to an integer). Incrementing is equivalent to adding one.
Non-Compliant Code Example
This code may result in a signed integer overflow during the addition of the signed operands si1
and si2
. If this behavior is unanticipated, it could lead to an exploitable vulnerability.
Code Block | ||
---|---|---|
| ||
| ||
Code Block | ||
| ||
int si1, si2, sum;
sum = si1 + si2;
|
...
Code Block | ||
---|---|---|
| ||
signed int si1, si2, sum; if ( ((si1^si2) | (((si1^(~(si1^si2) & (1 << (sizeof(int)*CHAR_BIT-1))))+si2)^si2)) >= 0) { /* handle error condition */ } sum = si1 + si2; |
Compliant Solution (General)
This compliant solution only works on architectures that use two's complement representation. While most modern platforms use two's complement representation, it is best not to introduce unnecessary platform dependencies when practical (see MSC14-A. Do not introduce unnecessary platform dependencies).
Compliant Solution (General)
This compliant solution tests the suspect addition operation to ensure no This compliant solution tests the suspect addition operation to ensure no overflow occurs regardless of representation.
...
Subtraction is between two operands of arithmetic type, two pointers to qualified or unqualified versions of compatible object types, or between a pointer to an object type and an integer type. Decrementing is equivalent to subtracting one.
Non-Compliant Code Example
This code can result in a signed integer overflow during the subtraction of the signed operands si1
and si2
. If this behavior is unanticipated, the resulting value may be used to allocate insufficient memory for a subsequent operation or in some other manner that could lead to an exploitable vulnerability.
See ARR36-C. Do not subtract or compare two pointers that do not refer to the same array, ARR37-C. Do not add or subtract an integer to a pointer to a non-array object, and ARR38-C. Do not add or subtract an integer to a pointer if the resulting value does not refer to an element within the array for rules about pointer subtraction. Decrementing is equivalent to subtracting one.
Non-Compliant Code Example
This non-compliant code example can result in a signed integer overflow during the subtraction of the signed operands si1
and si2
. If this behavior is unanticipated, the resulting value may be used to allocate insufficient memory for a subsequent operation or in some other manner that could lead to an exploitable vulnerability.
Code Block | ||
---|---|---|
| ||
signed int si1, si2, result;
result = si1 | ||
Code Block | ||
| ||
signed int si1, si2, result;
result = si1 - si2;
|
Compliant Solution (Two's Complement)
...
Code Block | ||
---|---|---|
| ||
signed int si1, si2, result; if (((si1^si2) & (((si1 ^ ((si1^si2) & (1 << (sizeof(int)*CHAR_BIT-1))))-si2)^si2)-si2)^si2)) < 0) { /* handle error condition */ } result = si1 - si2; ) < 0) { /* handle error condition */ } result = si1 - si2; |
This compliant solution only works on architectures that use two's complement representation. While most modern platforms use two's complement representation, it is best not to introduce unnecessary platform dependencies when practical (see MSC14-A. Do not introduce unnecessary platform dependencies).
Anchor | ||||
---|---|---|---|---|
|
...
The left shift operator is between two operands of integer type.This next example needs to be signed.
Non-Compliant Code Example
This non-compliant code example can result in signed integer overflow.
Code Block | ||
---|---|---|
| ||
int si1, si2, sresult; sresult = si1 << si2; |
...