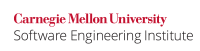
Dynamic memory management is a common source of programming flaws that can lead to security vulnerabilities. Decisions regarding how dynamic memory is allocated, used, and deallocated are the burden of the programmer. Poor memory management can lead to security issues, such as heap-buffer overflows, dangling pointers, and double-free issues [Seacord 2005a2013]. From the programmer's perspective, memory management involves allocating memory, reading and writing to memory, and deallocating memory.
...
Code Block | ||||
---|---|---|---|---|
| ||||
enum { MIN_SIZE_ALLOWED = 32 }; int verify_size(char *list, size_t size) { if (size < MIN_SIZE_ALLOWED) { /* Handle Errorerror Conditioncondition */ free(list); return -1; } return 0; } void process_list(size_t number) { char *list = (char *)malloc(number); if (list == NULL) { /* Handle Allocationallocation Errorerror */ } if (verify_size(list, number) == -1) { free(list); return; } /* Continue Processingprocessing list */ free(list); } |
The call to free memory in the verify_size()
function takes place in a subroutine of the process_list()
function, at a different level of abstraction from the allocation, resulting in a violation of this recommendation. The memory deallocation also occurs in error-handling code, which is frequently not as well tested as "green paths" through the code.
...
Code Block | ||||
---|---|---|---|---|
| ||||
enum { MIN_SIZE_ALLOWED = 32 }; int verify_size(const char *list, size_t size) { if (size < MIN_SIZE_ALLOWED) { /* Handle Errorerror Conditioncondition */ return -1; } return 0; } void process_list(size_t number) { char *list = (char *)malloc(number); if (list == NULL) { /* Handle Allocationallocation Errorerror */ } if (verify_size(list, number) == -1) { free(list); return; } /* Continue Processingprocessing list */ free(list); } |
Risk Assessment
...
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Compass/ROSE |
|
| Could detect possible violations by reporting any function that has | ||||||
Coverity | 6.5 | RESOURCE_LEAK | Fully Implemented. | ||||||
Fortify SCA | 5.0 |
| Can detect violations of this rule with CERT C Rule Pack. | ||||||
| 50 D | Partially implemented. |
...
[MIT 2004] | |
[Plakosh 2005] | |
[Seacord 2005a2013] | Chapter 4, "Dynamic Memory Management" |
...