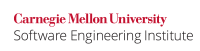
...
Include Page | ||||
---|---|---|---|---|
|
Non-Compliant Code Example
Type range errors, including loss of data (truncation) and loss of sign (sign errors), can occur when converting from an unsigned type to a signed type. The following code is likely to result in a truncation error for almost all implementations:
Code Block |
---|
unsigned long int ul = ULONG_MAX;
signed char sc;
sc = (signed char)ul; /* cast eliminates warning */
|
Compliant Solution
Validate ranges when converting from an unsigned type to a signed type. The following code, for example, can be used when converting from unsigned long int
to a signed char
.
Code Block |
---|
unsigned long int ul = ULONG_MAX; signed char sc; if (ul <= SCHAR_MAX) { sc = (signed char)ul; /* use cast to eliminate warning */ } else { /* handle error condition */ } |
Non-Compliant Code Example
Type range errors, including loss of data (truncation) and loss of sign (sign errors), can occur when converting from a signed type to an unsigned type. The following code results in a loss of sign:
...
NOTE: While unsigned types can usually represent all positive values of the corresponding signed type, this relationship is not guaranteed by the C99 standard.
Non-Compliant Code Example
A loss of data (truncation) can occur when converting from a signed type to a signed type with less precision. The following code is likely to result in a truncation error for most implementations:
...
Conversions from signed types with greater precision to signed types with lesser precision require both the upper and lower bounds to be checked.
Non-Compliant Code Example
A loss of data (truncation) can occur when converting from an unsigned type to an unsigned type with less precision. The following code is likely to result in a truncation error for most implementations:
...