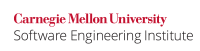
Prevent math errors by carefully bounds-checking before calling functions. In particular, the following domain errors should be prevented by prior bounds-checking:
...
Function | Bounds-checking |
---|
...
acos(x), |
...
asin(x) |
...
-1 |
...
<= |
...
x |
...
&& |
...
x |
...
<= |
...
1 |
...
atan2 | x != 0 || y != 0 |
log, log10 | x >= 0 |
pow(x, y) | x != 0 || y > 0 |
sqrt(x) | x >= 0 |
The calling function should take alternative action if these bounds are violated.
acos(x), asin(x)
Non-Compliant Example
The following code may produce a domain error if the argument is not in the range -1, +1.
Code Block |
---|
float x, result;
result = acos(x);
|
Compliant Example
The following code uses bounds checking to ensure there is not a domain error.
Code Block |
---|
float x, result;
if( x <= -1 || x >= 1){
/* handle domain error */
}
result = acos(x);
|
pow(x,y)
...
Non-Compliant Example
The following code may produce a domain error if x is zero and y less than or equal to zero. A range error may also occur if x is zero and y is negative.
...