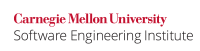
Wiki Markup |
---|
Dynamic memory managers are not required to clear freed memory and generally do not because of the additional runtime overhead. Furthermore, dynamic memory managers are free to reallocate this same memory. As a result, it is possible to accidently leak sensitive information if it is not cleared before calling a function which frees, or may free, dynamic memory. AAn example of this risk is the "Sun tarball" vulnerability described in \[Graff 03\]. Programmers cannot rely on memory being cleared during allocation either \[[MEM33-C. Do not assume memory allocation routines initialize memory]\]. |
Wiki Markup |
---|
In practice, this type of security flaw can expose sensitive information to unintended parties. The Sun tarball vulnerability discussed in _Secure Coding Principles & Practices: DesiginingDesigning and Implementing Secure Applications_ \[[Graf 03|AA. C References#Graf 03]\] and [Sun Security Bulletin #00122 | http://sunsolve.sun.com/search/document.do?assetkey=1-22-00122-1] illustrates a violation of this recommendation leading to sensitive data being leaked. Attackers may also be able to leverage this defect to retrieve sensitive information using techniques, such as _heap inspection_. |
...
Code Block | ||
---|---|---|
| ||
... char *new_secret; size_t size = strlen(secret); if (size == SIZE_MAX) { /* Handle Error */ } new_secret = calloc(size+1,sizeof(char)); /* use calloc() to zero-out allocated space */ if (!new_secret) { /* Handle Error */ } strcpy(new_secret, secret); /* Process new_secret... */ memset(new_secret, '\0', size); /* sanitize memory */ free(new_secret); ... |
...
Wiki Markup |
---|
Using {{realloc()}} to resize dynamic memory may inadvertently expose sensitive information, or allow heap inspection as is described in Fortify's Taxonomy of Software Security Errors \[[vulncat|http://vulncat.fortifysoftware.com/2/HI.html]\] and NIST's Source Code Analysis Tool Functional Specification \[[http://samate.nist.gov/docs/SAMATE_source_code_analysis_tool_spec_09_15_06.pdf]\]. When {{realloc()}} is called it may allocate a new, larger block of memory, copy the contents, of {{secret}} to this new block, {{free()}} the original block, and assign the newly allocated block to {{secret}}. However, the contents of the original block may remain in heap memory after being marked for deallocation. |
Code Block | ||
---|---|---|
| ||
... secret = realloc(secret, ,new_size); ... |
Compliant Solution: realloc()
...
Code Block | ||
---|---|---|
| ||
... temp_buff = calloc(new_size,sizeof(char)); /* use calloc() to zero-out allocated space */ if (temp_buff == NULL) { /* Handle Error */ } if (secret_size > new_size) /* may lead to truncation */ secret_size = new_size; memcpy(temp_buff, secret , secret_size); memset(secret, '\0', secret_size); /* sanitize the buffer */ free(secret); secret = temp_buff; /* install the resized buffer */ temp_buff = NULL; ... |
Wiki Markup |
---|
Note that this solution may truncate the contents of the original buffer, {{secret}}, if the size of the resized buffer is smaller. This behavior is similar to how {{realloc()}} handles resizing to a smaller block of memory \[[ISO/IEC 9899-1999|AA. C References#ISO/IEC 9899-1999]\]. |
...
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MEM33 MEM03-C A | 2 (medium) | 1 (unlikely) | 3 (low) | P6 | L2 |
...
- http://vulncat.fortifysoftware.com/2/HI.html
- http://samate.nist.gov/docs/SAMATE_source_code_analysis_tool_spec_09_15_06.pdf
- Graff 03 Graff, Mark G. and van Wyk, Kenneth R. Secure Coding Principles & Practices: Desigining Designing and Implementing Secure Applications. Sebastopol, CA: O'Reilly & Associates, 2003 (ISBN 0-596-00242-4).
- ISO/IEC 9899-1999 Section 7.20.3, Memory management functions