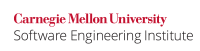
...
This example demonstrates an error that can occur when memory is freed in different functions. First, an array of integers is dynamically allocated. The array, which is referred to by list
and its size, number
, are then passed to func2
. If the number of elements in the array is greater than the value MIN_SIZE_ALLOWED
, the array is processed. Otherwise, it is assumed an error has occurred, list
is freed, and the function returns. If the error occurs in func2
, the dynamic memory referred to by list
will be freed twice: once in func2
and again at the end of func1
.
Code Block | ||
---|---|---|
| ||
#define MIN_SIZE_ALLOWED 10 void func2(int *list, size_t list_size) { if (size < MIN_SIZE_ALLOWED) { /* Handle Error Condition */ free(list); return; } /* Process list */ } void func1 (size_t number) { int *list = malloc (number * sizeof(int)); if (list == NULL) { /* Handle Allocation Error */ } func2(list,number); /* Continue Processing list */ free(list); } |
...
To correct this problem, the logic in the error handling code should be changed so that it no longer frees list
. This change ensures that list
is freed only once, in func1
.
Code Block | ||
---|---|---|
| ||
#define MIN_SIZE_ALLOWED 10 void func2(int *list, size_t list_size) { if (size < MIN_SIZE_ALLOWED) { /* Handle Error Condition */ return; } /* Process list */ } void func1 (size_t number) { int *list = malloc (number * sizeof(int)); if (list == NULL) { /* Handle Allocation Error */ } func2(list,number); /* Continue Processing list */ free(list); } |
...