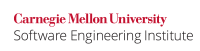
...
The gets()
function, which was deprecated in the C99 Technical Corrigendum 3 and removed from C11, is inherently unsafe and should never be used because it provides no way to control how much data is read into a buffer from stdin
. This noncompliant code example assumes that gets()
will not read more than BUFSIZ BUFFER_SIZE - 1
characters from stdin
. This is an invalid assumption, and the resulting operation can cause a buffer overflow. Note further that BUFSIZ
is a macro integer constant, defined in stdio.h, representing a suggested argument to setvbuf()
and not the maximum size of such an input buffer.
The gets()
function reads characters from the stdin
into a destination array until end-of-file is encountered or a newline character is read. Any newline character is discarded, and a null character is written immediately after the last character read into the array.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #define BUFFER_SIZE 1024 void func(void) { char buf[BUFSIZBUFFER_SIZE]; if (gets(buf) == NULL) { /* Handle error */ } } |
...
After the loop ends, if feof(stdin) != 0
, the loop has read through to the end of the file without encountering a newline character. Similarly, if ferror(stdin) != 0
, a read error occurred before the loop encountered a newline character, and if chars_read > index
, the input string has been truncated. FIO34-C. Do not compare Distinguish between characters read from a file with and EOF or WEOF is also applied in this solution.
...
...