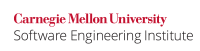
Modifying a variable through a pointer of an incompatible type (other than unsigned char
) can lead to unpredictable results. Subclause 6.2.7 of the C Standard mentions states that two types may be distinct yet compatible and addresses precisely when two distinct types are compatible.
This problem is often caused by a violation of aliasing rules. The C Standard, subclause 6.5, paragraph 7 [ISO/IEC 9899:2011], specifies those circumstances in which an object may or may not be aliased.
...
Accessing an object by means of any other lvalue expression (other than unsigned char
) results in is undefined behavior 37.
Noncompliant Code Example
In this noncompliant example, an object of type float
is incremented through an int *
. The programmer can use the unit in the last place can be used to get the next representable value for a floating-point type. However, accessing an object through a pointer of an incompatible types type is undefined behavior.
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> void f(void) { if (sizeof(int) == sizeof(float)) { float f = 0.0f; int *ip = (int *)&f; (*ip)++; printf("float is %f\n", f); } } |
...
In this compliant solution, the standard C function nextafterf()
is used to round towards toward the highest representable floating-point value:
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> void func(void) { union { short a[2]; int i; } u; u.a[0]=0x1111; u.a[1]=0x1111; u.i = 0x22222222; printf("%x %x\n", u.a[0], u.a[1]); /* ... */ } |
The printf()
behavior in this compliant solution is unspecified, but it is commonly accepted as an implementation extension (see unspecified behavior 11).
This function typically This code example now reliably outputs "2222 2222." However, there is no guarantee that this will be true, even on implementations that defined the unspecified behavior; values of type short
need not have the same representation as values of type int
.
Noncompliant Code Example
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h>
struct gadget {
int i;
double d;
char *p;
};
struct widget {
char *q;
int j;
double e;
};
void func(void) {
struct gadget *gp;
struct widget *wp;
gp = (struct gadget *)malloc(sizeof(struct gadget));
if (!gp) {
/* Handle error */
}
/* ... Initialize gadget ... */
wp = (struct widget *)realloc(gp, sizeof(struct widget));
if (!wp) {
free(gp);
/* Handle error */
}
if (wp->j == 12) {
/* ... */
}
} |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdlib.h> #include <string.h> struct gadget { int i; double d; char *p; }; struct widget { char *q; int j; double e; }; void func(void) { struct gadget *gp; struct widget *wp; gp = (struct gadget *)malloc(sizeof (struct gadget)); if (!gp) { /* Handle error */ } /* ... */ wp = (struct widget *)realloc(gp, sizeof(struct widget)); if (!wp) { free(gp); /* Handle error */ } memset(wp, 0, sizeof(struct widget)); /* ... Initialize widget ... */ if (wp->j == 12) { /* ... */ } } |
...
According to subclause 6.7.6.2 of the C Standard [ISO/IEC 9899:2011], using two or more incompatible arrays in an expression results in is undefined behavior. See (see also undefined behavior 76).
For two array types to be compatible, both should have compatible underlying element types, and both size specifiers should have the same constant value. If either of these properties is violated, the resulting behavior is undefined.
...
Optimizing for performance can lead to aliasing errors that can be quite difficult to detect. Furthermore, as in the preceding example, unexpected results can lead to buffer overflow attacks and/or , bypassing security checks and/, or unexpected execution.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
EXP39-C | Medium | Unlikely | High | P2 | L3 |
...
ISO/IEC TS 17961 | Accessing an object through a pointer to an incompatible type [ptrcomp] |
MITRE CWE | CWE-119, Failure to constrain operations Improper Restriction of Operations within the bounds of an allocated memory bufferBounds of a Memory Buffer |
Bibliography
[Acton 2006] | "Understanding Strict Aliasing" |
GCC Known Bugs | "C Bugs, Aliasing Issues while Casting to Incompatible Types" |
GCC Manual | |
[ISO/IEC 9899:2011] | Subclause 6.5, "Expressions" Subclause 6.7.6.2, "Array Declarators" |
[Walfridsson 2003] | Aliasing, Pointer Casts and GCC 3.3 |
...