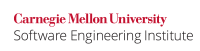
Pseudorandom number generators use mathematical algorithms to produce a sequence of numbers with good statistical properties, but the numbers produced are not genuinely random.
The C Standard function rand()
function makes no guarantees as to the quality of the random sequence produced. The numbers generated by some implementations of rand()
have a comparatively short cycle, and the numbers can be predictable. Applications that have strong pseudorandom number requirements should use a generator that is known to be sufficient for their needs.
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> void func(void) { enum { len = 12 }; char id[len]; /* * id will hold the ID, starting with the characters * the characters "ID" followed by a * random integer. */ */char id[len]; int r; int num; /* ... */ r = rand(); /* Generate a random integer */ num = snprintf(id, len, "ID%-d", r); /* Generate the ID */ /* ... */ } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <stdio.h> #include <stdlib.h> #include <time.h> void func(void) { enum { len = 12 }; char id[len]; /* * id will hold the ID, starting with the characters * the characters "ID" followed by a * random integer. */ */char id[len]; int r; int num; /* ... */ time_t now = time(NULL); if (now == (time_t)-1) { /* Handle error */ } srandom(now); /* Seed the PRNG with the current time */ /* ... */ r = random(); /* Generate a random integer */ num = snprintf(id, len, "ID%-d", r); /* Generate the ID */ /* ... */ } |
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <Windows.h> #include <wincrypt.h> #include <stdio.h> void func(void) { HCRYPTPROV prov; if (CryptAcquireContext(&prov, NULL, NULL, PROV_RSA_FULL, 0)) { long int li = 0; if (CryptGenRandom(prov, sizeof(li), (BYTE *)&li)) printf("Random number: %ld\n", li); CryptReleaseContext(prov, 0); } } |
Risk Assessment
Using The use of the rand()
function leads to possibly may result in predictable random numbers.
...