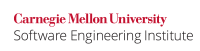
The calloc() function takes two arguments: the number of elements to allocate and the storage size of those elements. calloc() multiples these arguments together, and uses the result to specify the amount of memory to allocate. However, if the result of multiplying the number of elements to allocate and the storage size cannot be represented properly by an unsigned int, an integer overflow will occur. Therefore it is necessary to check the product of the arguments to calloc() for an integer overflow. If an overflow occurs, the program should detect and handle it appropriately.
Non-compliant Code Example 1
In this example, the user defined function get_size (not shown) is used to calculate the size requirements for a dynamic array of unsigned long long integers and stored in the variable num_elements. When calloc() is called to allocate the buffer, num_elements will be multiplied with the sizeof(unsigned long long) to compute the overall size requirements. If the value returned by get_size() is too large to be multiplied by sizeof(unsigned long long) and properly stored in an intermediate location within calloc(), then calloc() may allocate a buffer of insufficient size. When data is copied to that buffer, a buffer overflow may occur.
Code Block |
---|
size_t num_elements = get_size(); unsigned long long *buffer = calloc(num_elements, sizeof(unsigned long long)); |
Compliant Solution 1
Code Block |
---|
size_t num_elements = calc_size(); size_t size = sizeof(unsigned long long); if () { unsigned long long *buffer = calloc(num_elements, sizeof(unsigned long long)); } else { /* Handle error condition */ } |
References
RUS-CERT Advisory 2002-08:02, http://cert.uni-stuttgart.de/advisories/calloc.php
Secunia Advisory SA10635, http://secunia.com/advisories/10635/