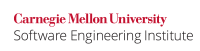
...
Code Block |
---|
char *copy(size_t n, char *str) { int i; char *p = malloc(n); for ( i = 0; i < n; ++i ) { p[i] = *str++; } return p; } char *p = copy(20, "hi there"); |
Compliant
...
Solution 1
Declaring i to be of type size_teliminates the possible integer overflow condition.
Code Block |
---|
char *copy(size_t n, char *str) {
size_t i;
char *p = malloc(n);
for ( i = 0; i < n; ++i ) {
p[i] = *str++;
}
return p;
}
char *p = copy(20, "hi there");
|
Non-compliant Code Example 2
The user defined function calc_size
(not shown) used to calculate the size of the string other_srting
. The result of calc_size
is a signed int
returned into str_size
. Given that there is no check on str_size
, it is impossible to tell whether the result of calc_size
is an appropriate parameter for malloc that is, a positive integer that can be properly represented by a signed int
type.
Code Block |
---|
int str_size = calc_size(other_string);
char *str_copy = malloc(str_size);
|
Compliant Code Example 2
By changing str_size
to a variable of type size_t
, it can be assured that the call to malloc()
is, at the least, supplied a non-negative number.
Code Block |
---|
size_t str_size = calc_size(other_string);
char *str_copy = malloc(str_size);
|
Non-compliant Code Example 2
Add an example using size_t as an index
References
- ISO/IEC 9899-1999 Section 7.17 Common definitions <stddef.h>
- ISO/IEC 9899-1999 Section 7.20.3 Memory management functions