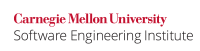
...
In this example, a file is opened for reading. If the file is opened successfully, memory is allocated by malloc()
and referenced by str
. A message indicating that the file was opened properly is copied into the dynamically-allocated memory referenced by str
and printed. Afterwards, the memory is deallocted by calling free()}
. If the file does not open correctly, however, {{str
is set to a string literal. Because str
now references memory that was not dynamically allocated, an error will occur when this memory is freed.
Code Block |
---|
FILE *file = NULL; char *str = NULL, *fname="~/config_file"; size_t size = 100; file = fopen("~/config_file","r"); if (file != NULL) { str = (char *)malloc(size); if (str == NULL) { /* Handle Allocation Error */ } snprintf(str, size, "File %s opened properly", fname); printf("LOG: %s\n", str); } else { str = "ERROR OPENING FILE"; printf("LOG: %s\n", str); } free(str); |
Compliant Solution 1
In the compliant solution, the call to free()
has been moved inside the conditional statement to ensure that only dynamic memory is freed.
Code Block |
---|
FILE *file = NULL; char *str = NULL, *fname="~/config_file"; size_t size = 100; file = fopen("~/config_file","r"); if (file != NULL) { str = (char *)malloc(size); if (str == NULL) { /* Handle Allocation Error */ } snprintf(str, size, "File %s opened properly", fname); printf("LOG: %s\n", str); free(str); /* only dynamic memory is freed */ } else { str = "ERROR OPENING FILE"; printf("LOG: %s\n", str); } |
Non-compliant Code Example 2
...