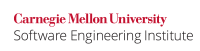
...
Non-compliant Code Example 2
This example attempts to resize the string referenced by buf
to make enough room to append the string line
. However, once in the function append()
, there is no way to determine how buf
was allocated. When realloc()
is called on buf
, since buf
does not point to dynamic memory, an error may occur.demonstrates an error that can occur when memory is freed in different functions. First, an array of number
integers is dynamically allocated. That array, referred to by list
, and its size, number
, are then passed to func2
. If the number of elements in the array is greater than the value MIN_SIZE_ALLOWED
, the array is processed. Otherwise, it is assumed an error has occurred, list
is freed, and the function returns. If the error occurs in func2
the dynamic memory referred to by list
will be freed twice: once in func2
and again at the end of func1
.
Code Block |
---|
#define MIN_SIZE_ALLOWED 10
void func2(int *list, size_t list_size) {
if (size < MIN_SIZE_ALLOWED) {
/* Error Condition */ |
Code Block |
void handle_error (char *buf) { printf("%s is not correct!\n",buf); free(buflist); return; } void func(char * str1, char * str2) { /* Process list */ } int func1 (size_t number) { int *list = malloc (number * sizeof(int)); if (list == NULL) { /* Handle Allocation Error */ } func2(list,number); /* Process list */ free(list); } |
Compliant Solution 2
Code Block |
---|
/* NOTE: buf must point to dynamically allocated memory */ void append(char \*buf, size_t count, size_t size) { char *line = " <- THIS IS A LINE"; size_t line_len = strlen(line); if ((count + line_len) > size) buf = realloc(buf,count+line_len); strncat(buf,line); } |
...