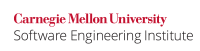
...
The
...
calloc()
...
function
...
takes
...
two
...
arguments:
...
the
...
number
...
of
...
elements
...
to
...
allocate
...
and
...
the
...
storage
...
size
...
of
...
those
...
elements.
...
The
...
calloc()
...
function
...
multiples
...
these
...
arguments
...
together
...
and
...
allocates
...
the
...
resulting
...
quantity
...
of
...
memory.
...
However,
...
if
...
the
...
result
...
of
...
multiplying
...
the
...
number
...
of
...
elements
...
to
...
allocate
...
and
...
the
...
storage
...
size
...
cannot
...
be
...
represented
...
properly
...
as
...
a
...
size_t
...
,
...
an
...
arithmetic
...
overflow
...
will
...
occur.
...
Therefore,
...
it
...
is
...
necessary
...
to
...
check
...
the
...
product
...
of
...
the
...
arguments
...
to
...
calloc()
...
for
...
an
...
arithmetic
...
overflow.
...
If
...
an
...
overflow
...
occurs,
...
the
...
program
...
should
...
detect
...
and
...
handle
...
it
...
appropriately.
...
Non-compliant
...
Code
...
Example
...
1
...
In
...
this
...
example,
...
the
...
user
...
defined
...
function
...
get_size()
...
(not
...
shown)
...
is
...
used
...
to
...
calculate
...
the
...
size
...
requirements
...
for
...
a
...
dynamic
...
array
...
of
...
unsigned
...
long
...
long
...
int
...
and
...
stored
...
in
...
the
...
variable
...
num_elements
...
.
...
When
...
calloc()
...
is
...
called
...
to
...
allocate
...
the
...
buffer,
...
num_elements
...
is
...
multiplied
...
by
...
the
...
sizeof(unsigned
...
long
...
long)
...
to
...
compute
...
the
...
overall
...
size
...
requirements.
...
If
...
the
...
number
...
of
...
elements
...
multiplied
...
by
...
the
...
size
...
can
...
not
...
be
...
represented
...
as
...
a
...
size_t
...
calloc()
...
may
...
allocate
...
a
...
buffer
...
of
...
insufficient
...
size.
...
When
...
data
...
is
...
copied
...
to
...
that
...
buffer,
...
a
...
buffer
...
overflow
...
may
...
occur.
Code Block |
---|
} size_t num_elements = get_size(); long *buffer = calloc(num_elements, sizeof(long)); if (buffer == NULL) { /* handle error condition */ } {code} h2. Compliant Solution 1 To correct this, a test is performed on the product of {{num_elements}} and {{sizeof(long)}} before the call to {{calloc()}}. The {{ |
Compliant Solution 1
To correct this, a test is performed on the product of num_elements
and sizeof(long)
before the call to calloc()
. The multsize_t()
...
function
...
sets
...
errno
...
to
...
a
...
non-zero
...
value
...
if
...
the
...
multiplication
...
operation
...
overflows.
Code Block |
---|
} long *buffer; size_t num_elements = calc_size(); (void) multsize_t(num_elements, sizeof(long)); if (errno) { /* handle error condition */ } buffer = calloc(num_elements, sizeof(long)); if (buffer == NULL) { /* handle error condition */ } {code} |
Note
...
that
...
the
...
maximum
...
amount
...
of
...
allocatable
...
memory
...
is
...
typically
...
limited
...
to
...
a
...
value
...
less
...
than
...
SIZE_MAX
...
(the
...
maximum
...
value
...
of
...
size_t
...
).
...
Always
...
check
...
the
...
return
...
value
...
from
...
a
...
call
...
to
...
any
...
memory
...
allocation
...
function.
...
References
...
- 7.18.3
...
- Limits
...
- of
...
- other
...
- integer
...
- types
...
- Seacord 05 Chapter 4 Dynamic Memory Management
- RUS-CERT
...
- Advisory
...
- 2002-08:02
...
...
- Secunia
...
- Advisory
...
- SA10635
...
...