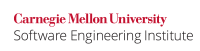
...
The following code will result in an unsigned integer overflow during the addition of the unsigned operands ui1 and ui2. If this behavior is unanticipated, the resulting value may be used to allocate insufficient memory for a subsequent operation or in some other manner which could lead to an exploitable vulnerability.
Code Block |
---|
unsigned int sum; unsigned int ui1 = UINT_MAX; unsigned int ui2 = 1, ui2, sum; sum = ui1 + ui2; |
Compliant Solution
...
Code Block |
---|
unsigned int ui1, ui2, sum;
if (~ui1 < ui2) {
error_handler("Overflow Error", NULL, EOVERFLOW);
}
sum = ui1 + ui2;
|
...
Code Block |
---|
signed int si1, si2, result;
if (si1 > 0){ /* si1 is positive */
if (si2 > 0) { /* si1 and si2 are positive */
if (si1 > (INT_MAX / si2)) {
error_handler("OVERFLOW ERROR", NULL, EOVERFLOW);
}
} /* end if si1 and si2 are positive */
else { /* si1 positive, si2 non-positive */
if (si2 < (INT_MIN / si1)) {
error_handler("OVERFLOW ERROR", NULL, EOVERFLOW);
}
} /* si1 positive, si2 non-positive */
} /* end if si1 is positive */
else { /* si1 is non-positive */
if (si2 > 0) { /* si1 is non-positive, si2 is positive */
if (si1 < (INT_MIN / si2)) {
error_handler("OVERFLOW ERROR", NULL, EOVERFLOW);
}
} /* end if si1 is non-positive, si2 is positive */
else { /* si1 and si2 are non-positive */
if( (si1 != 0) && (si2 < (INT_MAX / si1))) {
error_handler("OVERFLOW ERROR", NULL, EOVERFLOW);
}
} /* end if si1 and si2 are non-positive */
} /* end if si1 is non-positive */
result = si1 * si2;
|
...
Code Block |
---|
signed long sl1 = LONG_MIN; signed long sl2 = -1; signed long , sl2, result; result = sl1 / sl2; |
...
Code Block |
---|
signed long sl1 = LONG_MIN; signed long sl2 = -1; signed long , sl2, result; if ( (sl2 == 0) || ( (sl1 == LONG_MIN) && (sl2 == -1) ) ) { error_handler("ERROR OVERFLOW", NULL, EOVERFLOW); return 0; } result = sl1 / sl2; |
Modulo
...
Code Block |
---|
signed long sl1 = LONG_MIN; signed long sl2 = 0; signed long , sl2, result; result = sl1 % sl2; |
...
Code Block |
---|
signed long sl1 = LONG_MIN; signed long sl2 = 0; signed long , sl2, result; if ( sl2 == 0) { error_handler("ERROR OVERFLOW", NULL, EOVERFLOW); return 0; } result = sl1 % sl2; |
Unary Negation
...
The following code will result in a signed integer overflow during the unary negation of the signed operand si1. If this behavior is unanticipated, the resulting value may be used to allocate insufficient memory for a subsequent operation or in some other manner which could lead to an exploitable vulnerability.
Code Block |
---|
signed int result; signed int si1, = INT_MINresult; result = -si1; |
Compliant Solution
The following compliant solution tests the suspect negation operation to guarantee there is no possibility of signed overflow. In this particular case, an overflow condition is present and the error_handler()
method is invoked.
Code Block |
---|
signed int result; signed int si1, = INT_MINresult; if (si1 == INT_MIN) { error_handler("OVERFLOW ERROR", NULL, EOVERFLOW); return} result = -si1; |
Left Shift Operator
The shift operator in C is between two operands of integer type.
...
The following code will result in an unsigned overflow during the shift operation of the unsigned operands ui1
and ui2
. If this behavior is unanticipated, the resulting value may be used to allocate insufficient memory for a subsequent operation or in some other manner which could lead to an exploitable vulnerability.
Code Block |
---|
unsigned int result; unsigned int ui1 = UINT_MAX; unsigned int ui2 = sizeof(int)*8ui1, ui2, result; result = ui1 << ui2; |
Compliant Solution
The following compliant solution tests the suspect shift operation to guarantee there is no possibility of unsigned overflow. In this particular case, an overflow condition is present and the error_handler()
method is invoked.
Code Block |
---|
unsigned int result; unsigned int ui1 = UINT_MAX; unsigned int ui2 = sizeof(int)*8, ui2, result; if ( (ui2 < 0) || (ui2 >= sizeof(int)*8) ) { error_handler("OVERFLOW ERROR", NULL, EINVAL); } returnresult = ui1 << ui2; |
Right Shift Operator
...
The following code will result in an unsigned overflow during the shift operation of the unsigned operands ui1
and ui2
. If this behavior is unanticipated, the resulting value may be used to allocate insufficient memory for a subsequent operation or in some other manner which could lead to an exploitable vulnerability.
Code Block |
---|
unsigned int result; unsigned int ui1 = UINT_MAX; unsigned int ui2 = sizeof(int)*8ui1, ui2, result; result = ui1 >> ui2; |
Compliant Solution
The following compliant solution tests the suspect shift operation to guarantee there is no possibility of unsigned overflow. In this particular case, an overflow condition is present and the error_handler()
method is invoked.
Code Block |
---|
unsigned int result; unsigned int ui1 = UINT_MAX; unsigned int ui2 = sizeof(int)*8ui1, ui2, result; if ( (ui2 < 0) || (ui2 >= sizeof(int)*8) ) { error_handler("OVERFLOW ERROR", NULL, EINVAL); } returnresult = ui1 >> ui2; |