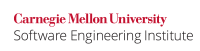
...
Operator | Overflow |
| Operator | Overflow |
| Operator | Overflow |
| Operator | Overflow |
---|---|---|---|---|---|---|---|---|---|---|
+ | yes |
| -= | yes |
| << | yes |
| < | no |
- | yes |
| *= | yes |
| >> | yes |
| > | no |
* | yes |
| /= | yes |
| & | no |
| >= | no |
/ | yes |
| %= | yes |
| | | no |
| <= | no |
% | yes |
| << | yes |
| ^ | no |
| == | no |
++ | yes |
| >>= | yes |
| ~ | no |
| != | no |
- | yes |
| &= | no |
| ! | no |
| && | no |
= | no |
| |= | no |
| un + | no |
| || | no |
+= | yes |
| ^= | no |
| un - | yes |
| ?: | no |
The following sections examine specific operations that are susceptible to integer overflow.
Addition
Addition in C is between two operands of arithmetic type, or between a pointer to an object type and an integer type. (Incrementing is equivalent to adding one.)
...
Code Block |
---|
unsigned int ui1, ui2, sum; if (~ui1 < ui2) { /* handle error_handler("Overflow Error", NULL, EOVERFLOW); condition */ } sum = ui1 + ui2; |
Subtraction
...
Code Block |
---|
signed int si1, si2, result; if ( ((si1^si2)&((si1-si2)^si1)) >> (sizeof(int)*CHAR_BIT-1) ) { /* handle error_handler("OVERFLOW ERROR", NULL, EOVERFLOW); condition */ } result = si1 - si2; |
Multiplication
...
Code Block |
---|
signed int si1, si2, result; signed long long tmp = (signed long long)si1 * (signed long long)si2; /* * If the product cannot be repesented as a 32-bit integer * then handle as an error condition */ if ( (tmp > INT_MAX) || (tmp < INT_MIN) ) { /* Thehandle product cannot fit in a 32-bit int */ error_handler("OVERFLOW ERROR", NULL, EOVERFLOW); error condition */ } result = (int)tmp; |
It is important to note that the above The preceeding code is only compliant on systems where long long
is at least twice the size of int
. On systems where this does not hold the following compliant solution may be used to ensure signed overflow does not occur.
...