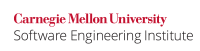
...
Code Block |
---|
signed int si1, si2, result; if (si1 > 0){ /* si1 is positive */ if (si2 > 0) { /* si1 and si2 are positive */ if (si1 > (INT_MAX / si2)) { error_handler("OVERFLOW ERROR", NULL, EOVERFLOW);/* handle error condition */ } } /* end if si1 and si2 are positive */ else { /* si1 positive, si2 non-positive */ if (si2 < (INT_MIN / si1)) { error_handler("OVERFLOW ERROR", NULL, EOVERFLOW);/* handle error condition */ } } /* si1 positive, si2 non-positive */ } /* end if si1 is positive */ else { /* si1 is non-positive */ if (si2 > 0) { /* si1 is non-positive, si2 is positive */ if (si1 < (INT_MIN / si2)) { error_handler("OVERFLOW ERROR", NULL, EOVERFLOW);/* handle error condition */ } } /* end if si1 is non-positive, si2 is positive */ else { /* si1 and si2 are non-positive */ if( (si1 != 0) && (si2 < (INT_MAX / si1))) { /* handle error_handler("OVERFLOW ERROR", NULL, EOVERFLOW); condition */ } } /* end if si1 and si2 are non-positive */ } /* end if si1 is non-positive */ result = si1 * si2; |
...
The following compliant solution tests the suspect division operation to guarantee there is no possibility of signed overflow. In this particular case, an overflow condition is present and the error_handler()
method is invoked.
Code Block |
---|
signed long sl1, sl2, result; if ( (sl2 == 0) || ( (sl1 == LONG_MIN) && (sl2 == -1) ) ) { /* handle error_handler("ERROR OVERFLOW", NULL, EOVERFLOW); condition */ } result = sl1 / sl2; |
Modulo
...
The following compliant solution tests the suspect Modulo operation to guarantee there is no possibility of signed overflow. In this particular case, an overflow condition is present and the error_handler()
method is invoked.
Code Block |
---|
signed long sl1, sl2, result; if (sl2 == 0) { error_handler("ERROR OVERFLOW", NULL, EOVERFLOW); /* handle error condition */ } result = sl1 % sl2; |
Unary Negation
...
The following compliant solution tests the suspect negation operation to guarantee there is no possibility of signed overflow. In this particular case, an overflow condition is present and the error_handler()
method is invoked.
Code Block |
---|
signed int si1, result; if (si1 == INT_MIN) { /* handle error_handler("OVERFLOW ERROR", NULL, EOVERFLOW); condition */ } result = -si1; |
Left Shift Operator
...
Code Block |
---|
unsigned int ui1, ui2, result; if ( (ui2 < 0) || (ui2 >= sizeof(int)*8) ) { /* handle error_handler("OVERFLOW ERROR", NULL, EINVAL); condition */ } result = ui1 << ui2; |
Right Shift Operator
...
The following compliant solution tests the suspect shift operation to guarantee there is no possibility of unsigned overflow. In this particular case, an overflow condition is present and the error_handler()
method is invoked.
Code Block |
---|
unsigned int ui1, ui2, result; if ( (ui2 < 0) || (ui2 >= sizeof(int)*8) ) { /* handle error_handler("OVERFLOW ERROR", NULL, EINVAL); condition */ } result = ui1 >> ui2; |
Exceptions
Unsigned integers can be allowed to exhibit modulo behavior if and only if
- the variable declaration is clearly commented as supporting modulo behavior
- each operation on that integer is also clearly commented as supporting modulo behavior
- if the integer exhibiting modulo behavior contributes to the value of an integer not marked as exhibiting modulo behavior, the resulting integer must obey this rule.
Consequences
Integer overflow can lead to buffer overflows and the execution of arbitary code by an attacker.
References
- Seacord 05 Chapter 5 Integers
- Warren 02 Chapter 2 Basics