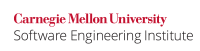
...
An alternative to invoking the system()
call to execute an external program to perform a required operation is to implement the functionality directly in the program using existing library calls. This compliant solution calls the POSIX
function to remove a file without invoking the unlink
()system()
function [IEEE Std 1003.1:2013]:
Code Block | ||||
---|---|---|---|---|
| ||||
#include <pwd.h> #include <unistd.h> #include <string.h> void func(void) { const char *file_format = "%s/.config"; size_t len; char *file; struct passwd *pwd; /* Get /etc/passwd entry for current user */ pwd = getpwuid(getuid()); if (pwd == NULL) { /* Handle error */ } /* Build full path name home dir from pw entry */ len = strlen(pwd->pw_dir) + strlen(file_format) + 1; file = (char *)malloc(len); if (NULL == file) { /* Handle error */ } int r = snprintf(file, len, file_format, pwd->pw_dir); if (r < 0 || r >= len) { /* Handle error */ } if (unlink(file) != 0) { /* Handle error */ } free(file); } |
The unlink()
function is not less susceptible to file
-related race conditions (see FIO01-C. Be careful using functions that use file names for identification) because if file
names a symbolic link, unlink()
will remove the symbolic link named by file
and not affect any file or directory named by the contents of the symbolic link. The fact that unlink()
operates on symbolic links only reduces its susceptibility to FIO01-C, it does not eliminate it. It is not susceptible as regards the final component of the pathname being a symbolic link, but it is still susceptible if the pathname contains a symbolic link in an earlier component, e.g. /tmp/symlink_to_dir/somefile
.
Compliant Solution (Windows)
...